http://www.tutorialspoint.com/xml-rpc/xml_rpc_examples.htm gives the below example:
import org.apache.xmlrpc.*;
public class JavaServer {
public Integer sum(int x, int y) {
return new Integer(x+y);
}
public static void main (String [] args) {
try {
System.out.println("Attempting to start XML-RPC Server...");
WebServer server = new WebServer(80);
server.addHandler("sample", new JavaServer());
server.start();
System.out.println("Started successfully.");
System.out.println("Accepting requests. (Halt program to stop.)");
} catch (Exception exception) {
System.err.println("JavaServer: " + exception);
}
}
}
------------------------------------
import java.util.*;
import org.apache.xmlrpc.*;
public class JavaClient {
public static void main (String [] args) {
try {
XmlRpcClient server = new XmlRpcClient("http://localhost:80");
Vector params = new Vector();
params.addElement(new Integer(17));
params.addElement(new Integer(13));
Object result = server.execute("sample.sum", params);
int sum = ((Integer) result).intValue();
System.out.println("The sum is: "+ sum);
} catch (Exception exception) {
System.err.println("JavaClient: " + exception);
}
}
}
This requires: xmlrpc-1.2.jar
in the Java build path. It works OK. No problem.
I will use this example to solve minor problems of 3 examples from
books.google.com - "Programming Web Services with XML-RPC of Simon Laurent, Joe Johnston,
and Edd Dumbill."
The problems that I met are:
1- Installing the helma.xmlrpc Library
You can not find this lib because it has evolved toxmlrpc.jar
2-the location of the handler
The examples are given in such fashion that the position of the handler
is left ambiguous. It should be an inner class of the server class.
3- the handler must be static
4- client-or the server does not need io try catch
5- Some examples require import for io
6- port problem connection error
The examples suggests a port number which does not connect at least on my machine.
I removed param processing which can easily be restored. I also
put the server and client into the same project for simplicity.
You should put them in seperate projects also.
An example of batch running follows:
1- XML-RPChandler project
C:\Users\ars\Desktop\eclipseWS\eclipseJAXWSrpc\XML-RPCproject\bin>java -cp ../li
b/xmlrpc-1.2.jar;. JavaServer
Attempting to start XML-RPC Server...
Started successfully.
Accepting requests. (Halt program to stop.)
C:\Users\ars\Desktop\eclipseWS\eclipseJAXWSrpc\XML-RPCproject\bin>java -cp ../li
b/xmlrpc-1.2.jar;. JavaClient
The sum is: 30
The corrected and running solutions lie below:
import java.io.IOException;
//import helma.xmlrpc.WebServer;
//import helma.xmlrpc.XmlRpc;
import org.apache.xmlrpc.*;
public class AreaServer {
public static class AreaHandler {
public Double rectArea(double length, double width) {
return new Double(length * width);
}
public Double circleArea(double radius) {
double value = (radius * radius * Math.PI);
return new Double(value);
}
}
public static void main(String[] args) {
// if (args.length < 1) {
// System.out.println(
// "Usage: java AreaServer [port]");
// System.exit(-1);
// }
// try {
// Start the server, using built-in version
System.out.println("Attempting to start XML-RPC Server...");
// WebServer server = new WebServer(Integer.parseInt(args[0]));
WebServer server = new WebServer(80);
System.out.println("Started successfully.");
// Register our handler class as area
//server.addHandler("area", new AreaHandler());
server.addHandler("area", new AreaHandler());
System.out.println("Registered AreaHandler class to area.");
server.start();
System.out.println("Started successfully.");
System.out.println("Now accepting requests. (Halt program to stop.)");
// } catch (IOException e) {
// System.out.println("Could not start server: " +
// e.getMessage( ));
// }
}
}
----------------------------------
import java.io.IOException;
import java.util.Vector;
//import helma.xmlrpc.XmlRpc;
//import helma.xmlrpc.XmlRpcClient;
//import helma.xmlrpc.XmlRpcException;
import org.apache.xmlrpc.*;
public class AreaClient {
public static void main(String args[]) {
// if (args.length < 1) {
// System.out.println(
// "Usage: java AreaClient [radius]");
// System.exit(-1);
// }
try {
// Create the client, identifying the server
XmlRpcClient client =
new XmlRpcClient("http://localhost:80/");
// Create the request parameters using user input
Vector params = new Vector( );
//params.addElement(new Double(args[0]));
params.addElement(new Double("5"));
// Issue a request
Object result =
client.execute("area.circleArea", params);
// Report the results
System.out.println("The area of the circle would be: " + result.toString( ));
} catch (IOException e) {
System.out.println("IO Exception: " + e.getMessage( ));
} catch (XmlRpcException e) {
System.out.println("Exception within XML-RPC: " + e.getMessage( ));
}
}
}
2.passing hash parameter example
import java.io.IOException;
import java.util.Hashtable;
//import helma.xmlrpc.WebServer;
//import helma.xmlrpc.XmlRpc;
import org.apache.xmlrpc.*;
public class AreaServer {
public static class AreaHandler {
public Double rectArea(double length, double width) {
return new Double(length * width);
}
public Double circleArea(double radius) {
double value = (radius * radius * Math.PI);
return new Double(value);
}
public Double anyArea(Hashtable arguments) {
Double value;
value=new Double(0);
String requestType=(String) arguments.get("type");
if (requestType.equals("circle")) {
Double radius=(Double) (arguments.get("radius"));
value=circleArea(radius.doubleValue( ));
}
if (requestType.equals("rectangle")) {
Double length=(Double) (arguments.get("length"));
Double width=(Double) (arguments.get("width"));
value=rectArea(length.doubleValue(), width.doubleValue( ));
}
return value;
}
}
public static void main(String[] args) {
// if (args.length < 1) {
// System.out.println(
// "Usage: java AreaServer [port]");
// System.exit(-1);
// }
// try {
// Start the server, using built-in version
System.out.println("Attempting to start XML-RPC Server...");
// WebServer server = new WebServer(Integer.parseInt(args[0]));
WebServer server = new WebServer(80);
System.out.println("Started successfully.");
// Register our handler class as area
//server.addHandler("area", new AreaHandler());
server.addHandler("area", new AreaHandler());
System.out.println("Registered AreaHandler class to area.");
server.start();
System.out.println("Started successfully.");
System.out.println("Now accepting requests. (Halt program to stop.)");
// } catch (IOException e) {
// System.out.println("Could not start server: " +
// e.getMessage( ));
// }
}
}
-------------------------------------------------
import java.io.IOException;
import java.util.Hashtable;
import java.util.Vector;
//import helma.xmlrpc.XmlRpc;
//import helma.xmlrpc.XmlRpcClient;
//import helma.xmlrpc.XmlRpcException;
import org.apache.xmlrpc.*;
public class AreaClient {
public static void main(String args[]) {
// if (args.length < 1) {
// System.out.println(
// "Usage: java AreaClient [radius]");
// System.exit(-1);
// }
try {
// Create the client, identifying the server
XmlRpcClient client =
new XmlRpcClient("http://localhost:80/");
// Create a double from the user argument
//Double radius=new Double(args[0]);
Double radius=new Double("5");
// Create a hashtable and add a circle request
Hashtable requestHash = new Hashtable( );
requestHash.put("type", "circle");
requestHash.put("radius", radius);
// Create the request parameters using user input
Vector params = new Vector( );
params.addElement(requestHash);
// Issue a request
Object result =
client.execute("area.anyArea", params);
// Report the results
System.out.println("The area of the circle would be: " + result.toString( ));
} catch (IOException e) {
System.out.println("IO Exception: " + e.getMessage( ));
} catch (XmlRpcException e) {
System.out.println("Exception within XML-RPC: " + e.getMessage( ));
}
}
}
3. log service program
import java.io.IOException;
//import helma.xmlrpc.WebServer;
//import helma.xmlrpc.XmlRpc;
import org.apache.xmlrpc.*;
public class XLogServer {
public static class XLogHandler {
public String XLogReport(String address, String message) {
System.out.println("From: " + address);
System.out.println("Message: " + message);
return "ack";
}
}
public static void main(String[] args) {
// if (args.length < 1) {
// System.out.println("Usage: java AreaServer [port]");
// System.exit(-1);
// }
// try {
// Start the server, using built-in version
System.out.println("Attempting to start XML-RPC Server...");
// WebServer server = new WebServer(Integer.parseInt(args[0]));
WebServer server = new WebServer(Integer.parseInt("80"));
System.out.println("Started successfully.");
// Register our handler class as area
server.addHandler("XLog", new XLogHandler());
System.out.println("Registered XLogHandler class to XLog.");
server.start();
System.out.println("Started successfully.");
System.out.println("Now accepting requests. (Halt program to stop.)");
// } catch (IOException e) {
// System.out.println("Could not start server: " +
// e.getMessage( ));
// }
}
}
-----------------------------------------------------------
import java.io.IOException;
import java.net.*;
import java.util.Vector;
//import helma.xmlrpc.XmlRpc;
//import helma.xmlrpc.XmlRpcClient;
//import helma.xmlrpc.XmlRpcException;
import org.apache.xmlrpc.*;
public class XLogClient {
public static void main(String args[]) {
try {
throw new Exception("help");
} catch (Exception e) {
report (e);
}
}
public static void report(Exception eReport) {
try {
// Create the client, identifying the server
XmlRpcClient client =
new XmlRpcClient("http://127.0.0.1:80/");
//new XmlRpcClient("http://192.168.2.191:80/");
//get local hostname and IP address
InetAddress address=InetAddress.getLocalHost( );
String ipAddress=address.toString( );
// Create the request parameters using user input
Vector params = new Vector( );
params.addElement(ipAddress);
params.addElement(eReport.getMessage( ));
// Issue a request
Object result =
client.execute("XLog.XLogReport", params);
// Report the results - this is just for the example
// In production, the 'ack' will be thrown away.
// Alternatively, the log system could be more interactive
// and the result might have meaning.
System.out.println("Response was: " + result.toString( ));
//If we can't report to server, report locally
} catch (IOException e) {
System.out.println("IO Exception: " + e.getMessage( ));
} catch (XmlRpcException e) {
System.out.println("Exception within XML-RPC: " + e.getMessage( ));
}
}
}
4. getting and setting a value
import java.io.IOException;
//import helma.xmlrpc.WebServer;
//import helma.xmlrpc.XmlRpc;
import org.apache.xmlrpc.*;
public class GetSetServer {
public static class GetSetHandler {
protected int value;
public GetSetHandler(int initialValue) {
value = initialValue;
}
public int getValue(String requester) {
return value;
}
public int setValue(String requester, int newValue) {
value = newValue;
return value;
}
}
public static void main(String[] args) {
// if (args.length < 1) {
// System.out.println(
// "Usage: java GetSetServer [port]");
// System.exit(-1);
// }
// try {
// Start the server, using built-in version
System.out.println("Attempting to start XML-RPC Server...");
// WebServer server = new WebServer(Integer.parseInt(args[0]));
WebServer server = new WebServer(Integer.parseInt("80"));
System.out.println("Started successfully.");
// Register our handler class as area
server.addHandler("getSet", new GetSetHandler(20));
System.out.println("Registered GetSetHandler class to getSet.");
server.start();
System.out.println("Started successfully.");
System.out.println("Now accepting requests. (Halt program to stop.)");
// } catch (IOException e) {
// System.out.println("Could not start server: " +
// e.getMessage( ));
// }
}
}
-------------------------------------------------
import java.io.IOException;
import java.net.*;
import java.util.Vector;
//import helma.xmlrpc.XmlRpc;
//import helma.xmlrpc.XmlRpcClient;
//import helma.xmlrpc.XmlRpcException;
import org.apache.xmlrpc.*;
public class GetSetClient {
public static void main(String args[]) {
if (args.length < 1) {
System.out.println(
"Usage: java GetSetClient [get set] [value]");
System.exit(-1);
}
String getOrSet=new String(args[0]);
if (!((getOrSet.equals("get")) (getOrSet.equals("set")))) {
System.out.println(
"First argument must be get or set");
System.exit(-1);
}
try {
// Create the client, identifying the server
XmlRpcClient client =
new XmlRpcClient("http://localhost:80/");
//get local host IP address
InetAddress address=InetAddress.getLocalHost( );
String ipAddress=address.toString( );
// Create the request parameters using user input
Vector params = new Vector( );
params.addElement(ipAddress);
if (getOrSet.equals("set")) {
Integer newValue=new Integer(args[1]);
params.addElement(newValue);
}
// Issue a request
Object result=null;
if (getOrSet.equals("set")) {
result = client.execute("getSet.setValue", params);
} else {
result = client.execute("getSet.getValue", params);
}
// Report the results
System.out.println("The response was: " + result.toString( ));
} catch (IOException e) {
System.out.println("IO Exception: " + e.getMessage( ));
} catch (XmlRpcException e) {
System.out.println("Exception within XML-RPC: " + e.getMessage( ));
}
}
}
Wednesday 28 March 2012
Converting JAX-WS examples from NB-GlassFish to Eclipse-GlassFish
This is a demo of converting JavaEE5-6 jaxws examples from NetBeans-GlassFish to Eclipse-GlassFish.
The difference occurs because of :
nlibraies.properties
libs.CopyLibs.classpath=\
${base}/CopyLibs/org-netbeans-modules-java-j2seproject-copylibstask.jar
libs.javaee-endorsed-api-6.0.classpath=\
${base}/javaee-endorsed-api-6.0/javax.annotation.jar;\
${base}/javaee-endorsed-api-6.0/jaxb-api-osgi.jar;\
${base}/javaee-endorsed-api-6.0/webservices-api-osgi.jar
libs.javaee-endorsed-api-6.0.javadoc=\
${base}/javaee-endorsed-api-6.0/javaee6-doc-api.zip
--------------------------------
C:\Users\ars\Desktop\ARSnbWebService\jaxws\helloservice\lib\javaee-endorsed-api-6.0
webservices-api-osgi.jar
jaxb-api-osgi.jar
javax.annotation
jaxrpc
saaj
wsdl4j
The NetBeans uses Metro Services or JAX-WS Reference Implementation which utilizes the above jars at the top. The Eclipse uses the above jar files and the AXIS implementation. So, there are structural differences in the implementation of JAX-WS.
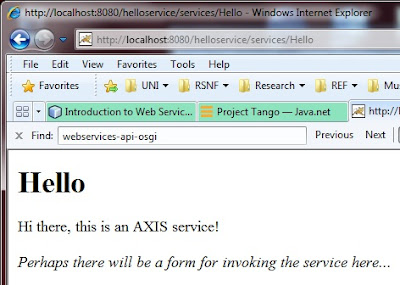
My solution for Eclipse-Tomcat conversion of the above Javaee5-6 example is:
You can find JAVAEE6 examples at http://docs.oracle.com/javaee/6/tutorial/doc/ (page 366).
The service part remains the same in Eclipse(page 368).
package helloservice.endpoint;
import javax.jws.WebService;
import javax.jws.webMethod;
@WebService
public class Hello {
private String message = new String("Hello, ");
public void Hello() {
}
@WebMethod
public String sayHello(String name) {
return message + name + ".";
}
}
But the client side causes problems when/if implemented as below:
package appclient;
import helloservice.endpoint.HelloService;
import javax.xml.ws.WebServiceRef;
public class HelloAppClient {
@WebServiceRef(wsdlLocation =
"META-INF/wsdl/localhost_8080/helloservice/HelloService.wsdl")
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
System.out.println(sayHello("world"));
}
private static String sayHello(java.lang.String arg0) {
helloservice.endpoint.Hello port = service.getHelloPort();
return port.sayHello(arg0);
}
}
I have two solutions to fix the problems at the client:
1- This is the same with the Tomcat solution.
/*
* Copyright 2011 Oracle and/or its affiliates.
* All rights reserved. You may not modify, use,
* reproduce, or distribute this software except in
* compliance with the terms of the License at:
* http://developers.sun.com/license/berkeley_license.html
*/
package webclient;
import helloservice.endpoint.*;
import java.io.IOException;
import java.io.PrintWriter;
import java.net.URL;
import java.rmi.RemoteException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.xml.namespace.QName;
import javax.xml.rpc.ServiceException;
import javax.xml.ws.Service;
import org.apache.axis.AxisFault;
import java.net.MalformedURLException;
import java.net.URL;
@WebServlet(name = "HelloServlet", urlPatterns = { "/HelloServlet" })
public class HelloServlet extends HttpServlet {
/**
* Processes requests for both HTTP <code>GET</code> and <code>POST</code>
* methods.
*
* @param request
* servlet request
* @param response
* servlet response
* @throws ServletException
* if a servlet-specific error occurs
* @throws IOException
* if an I/O error occurs
*/
protected void processRequest(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException, AxisFault ,Exception {
response.setContentType("text/html;charset=UTF-8");
PrintWriter out = response.getWriter();
try {
out.println("<html lang=\"en\">");
out.println("<head>");
out.println("<title>Servlet HelloServlet</title>");
out.println("</head>");
out.println("<body>");
out.println("<h1>Servlet HelloServlet at "
+ request.getContextPath() + "</h1>");
out.println("<p>" + sayHello("world") + "</p>");
out.println("</body>");
out.println("</html>");
} finally {
out.close();
}
}
// <editor-fold defaultstate="collapsed"
// desc="HttpServlet methods. Click on the + sign on the left to edit the code.">
/**
* Handles the HTTP <code>GET</code> method.
*
* @param request
* servlet request
* @param response
* servlet response
* @throws ServletException
* if a servlet-specific error occurs
* @throws IOException
* if an I/O error occurs
*/
@Override
protected void doGet(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
try{
processRequest(request, response);
} catch(AxisFault af){}
catch(Exception e){}
}
/**
* Handles the HTTP <code>POST</code> method.
*
* @param request
* servlet request
* @param response
* servlet response
* @throws ServletException
* if a servlet-specific error occurs
* @throws IOException
* if an I/O error occurs
*/
@Override
protected void doPost(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
try{
processRequest(request, response);
} catch(AxisFault af){}
catch(Exception e){}
}
/**
* Returns a short description of the servlet.
*
* @return a String containing servlet description
*/
@Override
public String getServletInfo() {
return "Short description";
} // </editor-fold>
private String sayHello(java.lang.String arg0) throws AxisFault ,Exception {
try {
// URL wsdlDocumentLocation = null;
// try {
// wsdlDocumentLocation = new URL(
// "http://localhost:8080/helloservice/HelloService?wsdl");
// //"http://localhost:8080/helloservice/services/Hello");
// } catch (MalformedURLException e) {
// e.printStackTrace();
// }
// QName qname = new QName("http://endpoint.helloservice/", "HelloService");
//
// Service service = Service.create(wsdlDocumentLocation, qname);
// System.out.println("aaaaaaaaaaaaa3");
// Hello hello = service.getPort(Hello.class);
// System.out.println("aaaaaaaaaaaaa4");
// return hello.sayHello(arg0);
// } catch (RemoteException re) {
// }
// return ("error");
//
// }
HelloSoapBindingStub srv = new HelloSoapBindingStub(
new URL("http://localhost:8080/helloservice/services/Hello"),
new HelloServiceLocator());
return srv.sayHello(arg0);
} catch(AxisFault af){}
return "error";
}
}
Please notice the changes that replace the commented code. I used a stub and its binding to the web service address and a locator to call the sayHello. The rest of the staff is pretty strqight forward but if any problems do not hesitate to contact me at arsaral(at)yahoo.com .
2- The client for GlassFish follows:
/*
* Copyright 2011 Oracle and/or its affiliates.
* All rights reserved. You may not modify, use,
* reproduce, or distribute this software except in
* compliance with the terms of the License at:
* http://developers.sun.com/license/berkeley_license.html
*/
package webclient;
import helloservice.endpoint.HelloService;
import helloservice.endpoint.*;
import java.io.IOException;
import java.io.PrintWriter;
import java.net.URL;
import java.rmi.RemoteException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.xml.namespace.QName;
import javax.xml.rpc.ServiceException;
import javax.xml.ws.Service;
import java.net.MalformedURLException;
import java.net.URL;
import com.sun.xml.ws.api.pipe.TransportPipeFactory;
@WebServlet(name = "HelloServlet", urlPatterns = { "/HelloServlet" })
public class HelloServlet extends HttpServlet {
/**
* Processes requests for both HTTP <code>GET</code> and <code>POST</code>
* methods.
*
* @param request
* servlet request
* @param response
* servlet response
* @throws ServletException
* if a servlet-specific error occurs
* @throws IOException
* if an I/O error occurs
*/
protected void processRequest(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html;charset=UTF-8");
PrintWriter out = response.getWriter();
try {
out.println("<html lang=\"en\">");
out.println("<head>");
out.println("<title>Servlet HelloServlet</title>");
out.println("</head>");
out.println("<body>");
out.println("<h1>Servlet HelloServlet at "
+ request.getContextPath() + "</h1>");
out.println("<p>" + sayHello("world") + "</p>");
out.println("</body>");
out.println("</html>");
} finally {
out.close();
}
}
// <editor-fold defaultstate="collapsed"
// desc="HttpServlet methods. Click on the + sign on the left to edit the code.">
/**
* Handles the HTTP <code>GET</code> method.
*
* @param request
* servlet request
* @param response
* servlet response
* @throws ServletException
* if a servlet-specific error occurs
* @throws IOException
* if an I/O error occurs
*/
@Override
protected void doGet(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
processRequest(request, response);
}
/**
* Handles the HTTP <code>POST</code> method.
*
* @param request
* servlet request
* @param response
* servlet response
* @throws ServletException
* if a servlet-specific error occurs
* @throws IOException
* if an I/O error occurs
*/
@Override
protected void doPost(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
processRequest(request, response);
}
/**
* Returns a short description of the servlet.
*
* @return a String containing servlet description
*/
@Override
public String getServletInfo() {
return "Short description";
} // </editor-fold>
private String sayHello(java.lang.String arg0) {
try {
URL wsdlDocumentLocation = null;
try {
wsdlDocumentLocation = new URL(
"http://localhost:8080/helloservice/HelloService?wsdl");
} catch (MalformedURLException e) {
e.printStackTrace();
}
QName qname = new QName("http://endpoint.helloservice/",
"HelloService");
Service service = Service.create(wsdlDocumentLocation, qname);
Hello hello = service.getPort(Hello.class);
return hello.sayHello(arg0);
} catch (RemoteException re) {
}
return ("error");
}
}
Please notice at the sayHello that Service class is used to get the service object using the wsdlDocumentLocation and qname. Be careful about the minor qname problem that is you write
QName qname = new QName("http://endpoint.helloservice/HelloService");
Instead of QName qname = new QName("http://endpoint.helloservice/","HelloService");
The compiler compiles it but gives the below error at the runtime:
javax.xml.ws.WebServiceException: {http://endpoint.helloservice/}HelloService is not a valid service. Valid services are: {http://endpoint.helloservice}HelloService
at com.sun.xml.ws.client.WSServiceDelegate.<init>(WSServiceDelegate.java:272)
at com.sun.xml.ws.client.WSServiceDelegate.<init>(WSServiceDelegate.java:205)
This is a demo of converting JavaEE5-6 jaxws examples from NetBeans-GlassFish to Eclipse-GlassFish.
The difference occurs because of :
nlibraies.properties
libs.CopyLibs.classpath=\
${base}/CopyLibs/org-netbeans-modules-java-j2seproject-copylibstask.jar
libs.javaee-endorsed-api-6.0.classpath=\
${base}/javaee-endorsed-api-6.0/javax.annotation.jar;\
${base}/javaee-endorsed-api-6.0/jaxb-api-osgi.jar;\
${base}/javaee-endorsed-api-6.0/webservices-api-osgi.jar
libs.javaee-endorsed-api-6.0.javadoc=\
${base}/javaee-endorsed-api-6.0/javaee6-doc-api.zip
--------------------------------
C:\Users\ars\Desktop\ARSnbWebService\jaxws\helloservice\lib\javaee-endorsed-api-6.0
webservices-api-osgi.jar
jaxb-api-osgi.jar
javax.annotation
jaxrpc
saaj
wsdl4j
The NetBeans uses Metro Services or JAX-WS Reference Implementation which utilizes the above jars at the top. The Eclipse uses the above jar files and the AXIS implementation. So, there are structural differences in the implementation of JAX-WS.
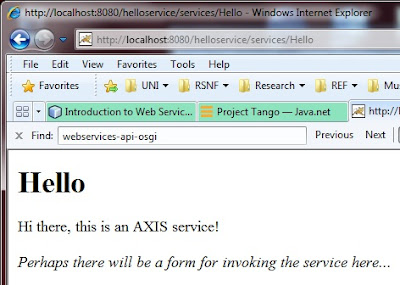
My solution for Eclipse-Tomcat conversion of the above Javaee5-6 example is:
You can find JAVAEE6 examples at http://docs.oracle.com/javaee/6/tutorial/doc/ (page 366).
The service part remains the same in Eclipse(page 368).
package helloservice.endpoint;
import javax.jws.WebService;
import javax.jws.webMethod;
@WebService
public class Hello {
private String message = new String("Hello, ");
public void Hello() {
}
@WebMethod
public String sayHello(String name) {
return message + name + ".";
}
}
But the client side causes problems when/if implemented as below:
package appclient;
import helloservice.endpoint.HelloService;
import javax.xml.ws.WebServiceRef;
public class HelloAppClient {
@WebServiceRef(wsdlLocation =
"META-INF/wsdl/localhost_8080/helloservice/HelloService.wsdl")
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
System.out.println(sayHello("world"));
}
private static String sayHello(java.lang.String arg0) {
helloservice.endpoint.Hello port = service.getHelloPort();
return port.sayHello(arg0);
}
}
I have two solutions to fix the problems at the client:
1- This is the same with the Tomcat solution.
/*
* Copyright 2011 Oracle and/or its affiliates.
* All rights reserved. You may not modify, use,
* reproduce, or distribute this software except in
* compliance with the terms of the License at:
* http://developers.sun.com/license/berkeley_license.html
*/
package webclient;
import helloservice.endpoint.*;
import java.io.IOException;
import java.io.PrintWriter;
import java.net.URL;
import java.rmi.RemoteException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.xml.namespace.QName;
import javax.xml.rpc.ServiceException;
import javax.xml.ws.Service;
import org.apache.axis.AxisFault;
import java.net.MalformedURLException;
import java.net.URL;
@WebServlet(name = "HelloServlet", urlPatterns = { "/HelloServlet" })
public class HelloServlet extends HttpServlet {
/**
* Processes requests for both HTTP <code>GET</code> and <code>POST</code>
* methods.
*
* @param request
* servlet request
* @param response
* servlet response
* @throws ServletException
* if a servlet-specific error occurs
* @throws IOException
* if an I/O error occurs
*/
protected void processRequest(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException, AxisFault ,Exception {
response.setContentType("text/html;charset=UTF-8");
PrintWriter out = response.getWriter();
try {
out.println("<html lang=\"en\">");
out.println("<head>");
out.println("<title>Servlet HelloServlet</title>");
out.println("</head>");
out.println("<body>");
out.println("<h1>Servlet HelloServlet at "
+ request.getContextPath() + "</h1>");
out.println("<p>" + sayHello("world") + "</p>");
out.println("</body>");
out.println("</html>");
} finally {
out.close();
}
}
// <editor-fold defaultstate="collapsed"
// desc="HttpServlet methods. Click on the + sign on the left to edit the code.">
/**
* Handles the HTTP <code>GET</code> method.
*
* @param request
* servlet request
* @param response
* servlet response
* @throws ServletException
* if a servlet-specific error occurs
* @throws IOException
* if an I/O error occurs
*/
@Override
protected void doGet(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
try{
processRequest(request, response);
} catch(AxisFault af){}
catch(Exception e){}
}
/**
* Handles the HTTP <code>POST</code> method.
*
* @param request
* servlet request
* @param response
* servlet response
* @throws ServletException
* if a servlet-specific error occurs
* @throws IOException
* if an I/O error occurs
*/
@Override
protected void doPost(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
try{
processRequest(request, response);
} catch(AxisFault af){}
catch(Exception e){}
}
/**
* Returns a short description of the servlet.
*
* @return a String containing servlet description
*/
@Override
public String getServletInfo() {
return "Short description";
} // </editor-fold>
private String sayHello(java.lang.String arg0) throws AxisFault ,Exception {
try {
// URL wsdlDocumentLocation = null;
// try {
// wsdlDocumentLocation = new URL(
// "http://localhost:8080/helloservice/HelloService?wsdl");
// //"http://localhost:8080/helloservice/services/Hello");
// } catch (MalformedURLException e) {
// e.printStackTrace();
// }
// QName qname = new QName("http://endpoint.helloservice/", "HelloService");
//
// Service service = Service.create(wsdlDocumentLocation, qname);
// System.out.println("aaaaaaaaaaaaa3");
// Hello hello = service.getPort(Hello.class);
// System.out.println("aaaaaaaaaaaaa4");
// return hello.sayHello(arg0);
// } catch (RemoteException re) {
// }
// return ("error");
//
// }
HelloSoapBindingStub srv = new HelloSoapBindingStub(
new URL("http://localhost:8080/helloservice/services/Hello"),
new HelloServiceLocator());
return srv.sayHello(arg0);
} catch(AxisFault af){}
return "error";
}
}
Please notice the changes that replace the commented code. I used a stub and its binding to the web service address and a locator to call the sayHello. The rest of the staff is pretty strqight forward but if any problems do not hesitate to contact me at arsaral(at)yahoo.com .
2- The client for GlassFish follows:
/*
* Copyright 2011 Oracle and/or its affiliates.
* All rights reserved. You may not modify, use,
* reproduce, or distribute this software except in
* compliance with the terms of the License at:
* http://developers.sun.com/license/berkeley_license.html
*/
package webclient;
import helloservice.endpoint.HelloService;
import helloservice.endpoint.*;
import java.io.IOException;
import java.io.PrintWriter;
import java.net.URL;
import java.rmi.RemoteException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.xml.namespace.QName;
import javax.xml.rpc.ServiceException;
import javax.xml.ws.Service;
import java.net.MalformedURLException;
import java.net.URL;
import com.sun.xml.ws.api.pipe.TransportPipeFactory;
@WebServlet(name = "HelloServlet", urlPatterns = { "/HelloServlet" })
public class HelloServlet extends HttpServlet {
/**
* Processes requests for both HTTP <code>GET</code> and <code>POST</code>
* methods.
*
* @param request
* servlet request
* @param response
* servlet response
* @throws ServletException
* if a servlet-specific error occurs
* @throws IOException
* if an I/O error occurs
*/
protected void processRequest(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html;charset=UTF-8");
PrintWriter out = response.getWriter();
try {
out.println("<html lang=\"en\">");
out.println("<head>");
out.println("<title>Servlet HelloServlet</title>");
out.println("</head>");
out.println("<body>");
out.println("<h1>Servlet HelloServlet at "
+ request.getContextPath() + "</h1>");
out.println("<p>" + sayHello("world") + "</p>");
out.println("</body>");
out.println("</html>");
} finally {
out.close();
}
}
// <editor-fold defaultstate="collapsed"
// desc="HttpServlet methods. Click on the + sign on the left to edit the code.">
/**
* Handles the HTTP <code>GET</code> method.
*
* @param request
* servlet request
* @param response
* servlet response
* @throws ServletException
* if a servlet-specific error occurs
* @throws IOException
* if an I/O error occurs
*/
@Override
protected void doGet(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
processRequest(request, response);
}
/**
* Handles the HTTP <code>POST</code> method.
*
* @param request
* servlet request
* @param response
* servlet response
* @throws ServletException
* if a servlet-specific error occurs
* @throws IOException
* if an I/O error occurs
*/
@Override
protected void doPost(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
processRequest(request, response);
}
/**
* Returns a short description of the servlet.
*
* @return a String containing servlet description
*/
@Override
public String getServletInfo() {
return "Short description";
} // </editor-fold>
private String sayHello(java.lang.String arg0) {
try {
URL wsdlDocumentLocation = null;
try {
wsdlDocumentLocation = new URL(
"http://localhost:8080/helloservice/HelloService?wsdl");
} catch (MalformedURLException e) {
e.printStackTrace();
}
QName qname = new QName("http://endpoint.helloservice/",
"HelloService");
Service service = Service.create(wsdlDocumentLocation, qname);
Hello hello = service.getPort(Hello.class);
return hello.sayHello(arg0);
} catch (RemoteException re) {
}
return ("error");
}
}
Please notice at the sayHello that Service class is used to get the service object using the wsdlDocumentLocation and qname. Be careful about the minor qname problem that is you write
QName qname = new QName("http://endpoint.helloservice/HelloService");
Instead of QName qname = new QName("http://endpoint.helloservice/","HelloService");
The compiler compiles it but gives the below error at the runtime:
javax.xml.ws.WebServiceException: {http://endpoint.helloservice/}HelloService is not a valid service. Valid services are: {http://endpoint.helloservice}HelloService
at com.sun.xml.ws.client.WSServiceDelegate.<init>(WSServiceDelegate.java:272)
at com.sun.xml.ws.client.WSServiceDelegate.<init>(WSServiceDelegate.java:205)
Tuesday 27 March 2012
Converting JAX-WS examples from NB-GlassFish to Eclipse-Tomcat
This is a demo of converting JavaEE5-6 jaxws examples from NetBeans-GlassFish to Eclipse-Tomcat and Eclipse-GlassFish.
You can find JAVAEE6 examples at http://docs.oracle.com/javaee/6/tutorial/doc/ (page 366).
The service part remains the same in Eclipse.
package helloservice.endpoint;
import javax.jws.WebService;
import javax.jws.webMethod;
@WebService
public class Hello {
private String message = new String("Hello, ");
public void Hello() {
}
@WebMethod
public String sayHello(String name) {
return message + name + ".";
}
}
But the client side causes problems when/if implemented as below(page 368).:
package appclient;
import helloservice.endpoint.HelloService;
import javax.xml.ws.WebServiceRef;
public class HelloAppClient {
@WebServiceRef(wsdlLocation =
"META-INF/wsdl/localhost_8080/helloservice/HelloService.wsdl")
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
System.out.println(sayHello("world"));
}
private static String sayHello(java.lang.String arg0) {
helloservice.endpoint.Hello port = service.getHelloPort();
return port.sayHello(arg0);
}
}
My Eclipse-TOMCAT version follows:
/*
* Copyright 2011 Oracle and/or its affiliates.
* All rights reserved. You may not modify, use,
* reproduce, or distribute this software except in
* compliance with the terms of the License at:
* http://developers.sun.com/license/berkeley_license.html
*/
package webclient;
import helloservice.endpoint.HelloService;
import java.io.IOException;
import java.io.PrintWriter;
import java.rmi.RemoteException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.xml.rpc.ServiceException;
import javax.xml.ws.WebServiceRef;
@WebServlet(name = "HelloServlet", urlPatterns = {"/HelloServlet"})
public class HelloServlet extends HttpServlet {
//@WebServiceRef(wsdlLocation = "WEB-INF/wsdl/ars-PC_8080/helloservice/HelloService.wsdl")
@WebServiceRef(wsdlLocation = "http:///ars-PC:8080/helloservice/HelloService?wsdl")
public HelloService service;
/**
* Processes requests for both HTTP <code>GET</code>
* and <code>POST</code> methods.
* @param request servlet request
* @param response servlet response
* @throws ServletException if a servlet-specific error occurs
* @throws IOException if an I/O error occurs
*/
protected void processRequest(
HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html;charset=UTF-8");
PrintWriter out = response.getWriter();
try {
out.println("<html lang=\"en\">");
out.println("<head>");
out.println("<title>Servlet HelloServlet</title>");
out.println("</head>");
out.println("<body>");
out.println(
"<h1>Servlet HelloServlet at " + request.getContextPath()
+ "</h1>");
out.println("<p>" + sayHello("world") + "</p>");
out.println("</body>");
out.println("</html>");
} finally {
out.close();
}
}
// <editor-fold defaultstate="collapsed" desc="HttpServlet methods. Click on the + sign on the left to edit the code.">
/**
* Handles the HTTP <code>GET</code> method.
* @param request servlet request
* @param response servlet response
* @throws ServletException if a servlet-specific error occurs
* @throws IOException if an I/O error occurs
*/
@Override
protected void doGet(
HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
processRequest(request, response);
}
/**
* Handles the HTTP <code>POST</code> method.
* @param request servlet request
* @param response servlet response
* @throws ServletException if a servlet-specific error occurs
* @throws IOException if an I/O error occurs
*/
@Override
protected void doPost(
HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
processRequest(request, response);
}
/**
* Returns a short description of the servlet.
* @return a String containing servlet description
*/
@Override
public String getServletInfo() {
return "Short description";
} // </editor-fold>
private String sayHello(java.lang.String arg0) {
try {
helloservice.endpoint.Hello port = service.getHello();
// port = service.getHelloPort();
return port.sayHello(arg0);
} catch(ServiceException e){}
catch(RemoteException re){};
return "error";
}
}
The problems I met during this effort:
javax.servlet.ServletException: Error instantiating servlet class webclient.HelloServlet
...
root cause
javax.naming.NameNotFoundException: Name webclient.HelloServlet is not bound in this Context
org.apache.naming.NamingContext.lookup(NamingContext.java:803)
org.apache.naming.NamingContext.lookup(NamingContext.java:159)
This error stops when I comment the @WebServiceRef line. But then the service.getHello() does not work due to the null service from the lack of injection.
Some other problems I met while dealing with this problem:
After
wsdlDocumentLocation = new URL(
// "http://localhost:8080/helloservice/HelloService?wsdl");
"http://localhost:8080/helloservice/services/Hello");
Mar 19, 2012 7:57:32 PM org.apache.catalina.core.StandardWrapperValve invoke
SEVERE: Servlet.service() for servlet [Hello] in context with path [/webclient] threw exception
javax.xml.ws.WebServiceException: {http://endpoint.helloservice/}HelloService is not a valid service. Valid services are: {http://endpoint.helloservice}HelloService
at com.sun.xml.ws.client.WSServiceDelegate.<init>(WSServiceDelegate.java:272)
at ...
I also got this at one point:
type Exception report
descriptionThe server encountered an internal error () that prevented it from fulfilling this request.
exception
com.sun.xml.ws.util.ServiceConfigurationError: com.sun.xml.ws.api.pipe.TransportPipeFactory: Provider com.sun.enterprise.jbi.serviceengine.bridge.transport.JBITransportPipeFactory is specified in bundle://293.0:0/META-INF/services/com.sun.xml.ws.api.pipe.TransportPipeFactory but not found
note The full stack traces of the exception and its root causes are available in the GlassFish Server Open Source Edition 3.1 logs.
The solution of the problem is:
nlibraies.properties
libs.CopyLibs.classpath=\
${base}/CopyLibs/org-netbeans-modules-java-j2seproject-copylibstask.jar
libs.javaee-endorsed-api-6.0.classpath=\
${base}/javaee-endorsed-api-6.0/javax.annotation.jar;\
${base}/javaee-endorsed-api-6.0/jaxb-api-osgi.jar;\
${base}/javaee-endorsed-api-6.0/webservices-api-osgi.jar
libs.javaee-endorsed-api-6.0.javadoc=\
${base}/javaee-endorsed-api-6.0/javaee6-doc-api.zip
--------------------------------
C:\Users\ars\Desktop\ARSnbWebService\jaxws\helloservice\lib\javaee-endorsed-api-6.0
webservices-api-osgi.jar
jaxb-api-osgi.jar
javax.annotation
jaxrpc
saaj
wsdl4j

My solution for Eclipse-Tomcat conversion of the above Javaee5-6 example is:
/*
* Copyright 2011 Oracle and/or its affiliates.
* All rights reserved. You may not modify, use,
* reproduce, or distribute this software except in
* compliance with the terms of the License at:
* http://developers.sun.com/license/berkeley_license.html
*/
package webclient;
import helloservice.endpoint.*;
import java.io.IOException;
import java.io.PrintWriter;
import java.net.URL;
import java.rmi.RemoteException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.xml.namespace.QName;
import javax.xml.rpc.ServiceException;
import javax.xml.ws.Service;
import org.apache.axis.AxisFault;
import java.net.MalformedURLException;
import java.net.URL;
@WebServlet(name = "HelloServlet", urlPatterns = { "/HelloServlet" })
public class HelloServlet extends HttpServlet {
/**
* Processes requests for both HTTP <code>GET</code> and <code>POST</code>
* methods.
*
* @param request
* servlet request
* @param response
* servlet response
* @throws ServletException
* if a servlet-specific error occurs
* @throws IOException
* if an I/O error occurs
*/
protected void processRequest(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException, AxisFault ,Exception {
response.setContentType("text/html;charset=UTF-8");
PrintWriter out = response.getWriter();
try {
out.println("<html lang=\"en\">");
out.println("<head>");
out.println("<title>Servlet HelloServlet</title>");
out.println("</head>");
out.println("<body>");
out.println("<h1>Servlet HelloServlet at "
+ request.getContextPath() + "</h1>");
out.println("<p>" + sayHello("world") + "</p>");
out.println("</body>");
out.println("</html>");
} finally {
out.close();
}
}
// <editor-fold defaultstate="collapsed"
// desc="HttpServlet methods. Click on the + sign on the left to edit the code.">
/**
* Handles the HTTP <code>GET</code> method.
*
* @param request
* servlet request
* @param response
* servlet response
* @throws ServletException
* if a servlet-specific error occurs
* @throws IOException
* if an I/O error occurs
*/
@Override
protected void doGet(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
try{
processRequest(request, response);
} catch(AxisFault af){}
catch(Exception e){}
}
/**
* Handles the HTTP <code>POST</code> method.
*
* @param request
* servlet request
* @param response
* servlet response
* @throws ServletException
* if a servlet-specific error occurs
* @throws IOException
* if an I/O error occurs
*/
@Override
protected void doPost(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
try{
processRequest(request, response);
} catch(AxisFault af){}
catch(Exception e){}
}
/**
* Returns a short description of the servlet.
*
* @return a String containing servlet description
*/
@Override
public String getServletInfo() {
return "Short description";
} // </editor-fold>
private String sayHello(java.lang.String arg0) throws AxisFault ,Exception {
try {
// URL wsdlDocumentLocation = null;
// try {
// wsdlDocumentLocation = new URL(
// "http://localhost:8080/helloservice/HelloService?wsdl");
// //"http://localhost:8080/helloservice/services/Hello");
// } catch (MalformedURLException e) {
// e.printStackTrace();
// }
// QName qname = new QName("http://endpoint.helloservice/", "HelloService");
//
// Service service = Service.create(wsdlDocumentLocation, qname);
// System.out.println("aaaaaaaaaaaaa3");
// Hello hello = service.getPort(Hello.class);
// System.out.println("aaaaaaaaaaaaa4");
// return hello.sayHello(arg0);
// } catch (RemoteException re) {
// }
// return ("error");
//
// }
HelloSoapBindingStub srv = new HelloSoapBindingStub(
new URL("http://localhost:8080/helloservice/services/Hello"),
new HelloServiceLocator());
return srv.sayHello(arg0);
} catch(AxisFault af){}
return "error";
}
}
Please notice the changes that replace the commented code. I used a stub and its binding to the web service address and a locator to call the sayHello. The rest of the staff is pretty straight forward but if any problems do not hesitate to contact me at arsaral(at)yahoo.com .
An example of Eclipse-GlassFish conversion of the NetBeans-GlassFish version will follow.
Wednesday 14 March 2012
An Analysis of JAX-WS usage of NetBeans2.2
Webclient Content Comparison of development phases
1. The comparison of the initial and wsimport phases. Wsimport phase includes only the creation of WSDL based connection to the webservice.
1.1 A new build folder is created at the project root although we have not done any build operation. This folder has:
webclient\build
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> generated
03/11/2012 07:24 PM <DIR> generated-sources
0 File(s) 0 bytes
As seen above two new folders named generrated and generated-sources are created in the new build folder. These folders contain the generated java files related with the webservice. Generated and generated-sources are exactly the same.
webclient\build\generated\jax-wsCache\HelloService\webclient has:
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:22 PM 1,751 Hello.java
03/11/2012 07:22 PM 745 HelloResponse.java
03/11/2012 07:22 PM 2,957 HelloService.java
03/11/2012 07:22 PM 718 Hello_Type.java
03/11/2012 07:22 PM 3,693 ObjectFactory.java
03/11/2012 07:22 PM 103 package-info.java
03/11/2012 07:22 PM 1,347 SayHello.java
03/11/2012 07:22 PM 1,481 SayHelloResponse.java
8 File(s) 12,795 bytes
webclient\build\generated-sources\jax-ws\webclient
...
8 File(s) 12,795 bytes
1.2 The nbproject folder has changed similar to the webservice creation phase. There is a new
Jaxws-build.xml and jax-ws.xml addition. But this time wsimport facilities are used in the jaxws-build.xml.
1.2.1 Project.xml
<?xml version="1.0" encoding="UTF-8" ?>
- <project xmlns="http://www.netbeans.org/ns/project/1">
<type>org.netbeans.modules.web.project</type>
- <configuration>
- <buildExtensions xmlns="http://www.netbeans.org/ns/ant-build-extender/1">
- <extension file="jaxws-build.xml" id="jaxws">
<dependency dependsOn="wsimport-client-generate" target="-pre-pre-compile" />
</extension>
</buildExtensions>
1.2.2 jax-ws.xml
<?xml version="1.0" encoding="UTF-8" ?>
- <jax-ws xmlns="http://www.netbeans.org/ns/jax-ws/1">
<services />
- <clients>
- <client name="HelloService">
<wsdl-url>http://ars-PC:8080/helloservice/HelloService?wsdl</wsdl-url>
<local-wsdl-file>ars-PC_8080/helloservice/HelloService.wsdl</local-wsdl-file>
<package-name forceReplace="true">webclient</package-name>
<catalog-file>catalog.xml</catalog-file>
- <wsimport-options>
- <wsimport-option>
<wsimport-option-name>extension</wsimport-option-name>
<wsimport-option-value>true</wsimport-option-value>
</wsimport-option>
- <wsimport-option>
<wsimport-option-name>verbose</wsimport-option-name>
<wsimport-option-value>true</wsimport-option-value>
</wsimport-option>
- <wsimport-option>
<wsimport-option-name>wsdlLocation</wsimport-option-name>
<wsimport-option-value>http://ars-PC:8080/helloservice/HelloService?wsdl</wsimport-option-value>
</wsimport-option>
- <wsimport-option>
<wsimport-option-name>xnocompile</wsimport-option-name>
<wsimport-option-value>true</wsimport-option-value>
</wsimport-option>
- <wsimport-option>
<wsimport-option-name>xendorsed</wsimport-option-name>
<wsimport-option-value>true</wsimport-option-value>
</wsimport-option>
- <wsimport-option>
<wsimport-option-name>package</wsimport-option-name>
<wsimport-option-value>webclient</wsimport-option-value>
</wsimport-option>
</wsimport-options>
</client>
</clients>
</jax-ws>
1.2.3 jaxws-build.xml
<?xml version="1.0" encoding="UTF-8" ?>
- <!--
*** GENERATED FROM jax-ws.xml - DO NOT EDIT ! ***
*** TO MODIFY wsimport options USE Web Service node -> Edit WS Attributes ***
*** TO CHANGE TARGETS GENERATED TO jaxws-build.xml COPY THOSE ***
*** TARGETS TO ../build.xml AND MODIFY THAT FILE INSTEAD ***
-->
- <project xmlns:xalan="http://xml.apache.org/xslt" xmlns:webproject2="http://www.netbeans.org/ns/web-project/2" xmlns:jaxws="http://www.netbeans.org/ns/jax-ws/1">
- <!--
===================
JAX-WS WSGEN SECTION
===================
-->
- <!--
===================
JAX-WS WSIMPORT SECTION
===================
-->
- <target name="wsimport-init" depends="init">
<mkdir dir="${build.generated.sources.dir}/jax-ws" />
<property name="j2ee.platform.wsimport.classpath" value="${libs.jaxws21.classpath}" />
- <taskdef name="wsimport" classname="com.sun.tools.ws.ant.WsImport">
<classpath path="${java.home}/../lib/tools.jar:${j2ee.platform.wsimport.classpath}" />
</taskdef>
- <condition property="conf-dir" value="${conf.dir}/" else="">
<isset property="conf.dir" />
</condition>
</target>
- <target name="wsimport-client-HelloService" depends="wsimport-init">
<mkdir dir="${build.generated.dir}/jax-wsCache/HelloService" />
- <wsimport sourcedestdir="${build.generated.dir}/jax-wsCache/HelloService" destdir="${build.generated.dir}/jax-wsCache/HelloService" wsdl="${basedir}/${conf-dir}xml-resources/web-service-references/HelloService/wsdl/ars-PC_8080/helloservice/HelloService.wsdl" catalog="catalog.xml" extension="true" verbose="true" wsdlLocation="http://ars-PC:8080/helloservice/HelloService?wsdl" xnocompile="true" xendorsed="true" package="webclient">
<depends file="${basedir}/${conf-dir}xml-resources/web-service-references/HelloService/wsdl/ars-PC_8080/helloservice/HelloService.wsdl" />
<produces dir="${build.generated.dir}/jax-wsCache/HelloService" />
</wsimport>
- <copy todir="${build.generated.sources.dir}/jax-ws">
- <fileset dir="${build.generated.dir}/jax-wsCache/HelloService">
<include name="**/*.java" />
</fileset>
</copy>
</target>
- <target name="wsimport-client-clean-HelloService" depends="-init-project">
<delete dir="${build.generated.sources.dir}/jax-ws/webclient" />
<delete dir="${build.generated.dir}/jax-wsCache/HelloService" />
</target>
<target name="wsimport-client-generate" depends="wsimport-client-HelloService" />
</project>
1.3 The last change is the xml-resources folder created newly in the /src/conf/ folder as seen below:
Directory of C:\Users\ars\Desktop\webclientARS\src\conf\xml-resources\web-service-references\HelloService\wsdl\ars-PC_8080\helloservice
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:22 PM 2,593 HelloService.wsdl
03/11/2012 07:22 PM 1,024 HelloService.xsd_1.xsd
2 File(s) 3,617 bytes
The folder above contains the WSDL files.
1.4 webclient\web\WEB-INF\wsdl\ars-PC_8080\helloservice folder also has the wsdl files:
HelloService.wsdl and HelloService.xsd_1.xsd
2. The comparison of the output of the build phase to the WS-IMPORT phase.
2.1 As expected the build phase creates the dist folder which contains the distribution WAR. It also creates the web folder in the build folder.
Directory of C:\Users\ars\Desktop\webclientARS\build\web\WEB-INF
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM <DIR> classes
03/11/2012 08:18 PM 521 glassfish-web.xml
03/11/2012 08:18 PM 411 jax-ws-catalog.xml
03/11/2012 08:18 PM <DIR> wsdl
2 File(s) 932 bytes
...
webclient\build\web\WEB-INF\classes\webclient
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM 1,183 Hello.class
03/11/2012 08:18 PM 507 HelloResponse.class
03/11/2012 08:18 PM 2,691 HelloService.class
03/11/2012 08:18 PM 2,611 HelloServlet.class
03/11/2012 08:18 PM 490 Hello_Type.class
03/11/2012 08:18 PM 2,778 ObjectFactory.class
03/11/2012 08:18 PM 244 package-info.class
03/11/2012 08:18 PM 757 SayHello.class
03/11/2012 08:18 PM 863 SayHelloResponse.class
9 File(s) 12,124 bytes
...
webclient\build\web\WEB-INF\wsdl\ars-PC_8080\helloservice
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM 2,593 HelloService.wsdl
03/11/2012 08:18 PM 1,024 HelloService.xsd_1.xsd
2 File(s) 3,617 bytes
Nbproject folder shows some changes at genfiles.properties, which may be trivial.
2.2 It creates an empty ap-source-output folder in the build/generated-sources folder.
3. The comparison of the outcome of the execution phase to the build phase in terms of the files’ and folders’ of the project follows: Please notice the lengths of Hello.class and HelloServlet.class which reside at webclient\build\web\WEB-INF\classes\webclient
have increased after running the client.
1. The comparison of the initial and wsimport phases. Wsimport phase includes only the creation of WSDL based connection to the webservice.
1.1 A new build folder is created at the project root although we have not done any build operation. This folder has:
webclient\build
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> generated
03/11/2012 07:24 PM <DIR> generated-sources
0 File(s) 0 bytes
As seen above two new folders named generrated and generated-sources are created in the new build folder. These folders contain the generated java files related with the webservice. Generated and generated-sources are exactly the same.
webclient\build\generated\jax-wsCache\HelloService\webclient has:
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:22 PM 1,751 Hello.java
03/11/2012 07:22 PM 745 HelloResponse.java
03/11/2012 07:22 PM 2,957 HelloService.java
03/11/2012 07:22 PM 718 Hello_Type.java
03/11/2012 07:22 PM 3,693 ObjectFactory.java
03/11/2012 07:22 PM 103 package-info.java
03/11/2012 07:22 PM 1,347 SayHello.java
03/11/2012 07:22 PM 1,481 SayHelloResponse.java
8 File(s) 12,795 bytes
webclient\build\generated-sources\jax-ws\webclient
...
8 File(s) 12,795 bytes
1.2 The nbproject folder has changed similar to the webservice creation phase. There is a new
Jaxws-build.xml and jax-ws.xml addition. But this time wsimport facilities are used in the jaxws-build.xml.
1.2.1 Project.xml
<?xml version="1.0" encoding="UTF-8" ?>
- <project xmlns="http://www.netbeans.org/ns/project/1">
<type>org.netbeans.modules.web.project</type>
- <configuration>
- <buildExtensions xmlns="http://www.netbeans.org/ns/ant-build-extender/1">
- <extension file="jaxws-build.xml" id="jaxws">
<dependency dependsOn="wsimport-client-generate" target="-pre-pre-compile" />
</extension>
</buildExtensions>
1.2.2 jax-ws.xml
<?xml version="1.0" encoding="UTF-8" ?>
- <jax-ws xmlns="http://www.netbeans.org/ns/jax-ws/1">
<services />
- <clients>
- <client name="HelloService">
<wsdl-url>http://ars-PC:8080/helloservice/HelloService?wsdl</wsdl-url>
<local-wsdl-file>ars-PC_8080/helloservice/HelloService.wsdl</local-wsdl-file>
<package-name forceReplace="true">webclient</package-name>
<catalog-file>catalog.xml</catalog-file>
- <wsimport-options>
- <wsimport-option>
<wsimport-option-name>extension</wsimport-option-name>
<wsimport-option-value>true</wsimport-option-value>
</wsimport-option>
- <wsimport-option>
<wsimport-option-name>verbose</wsimport-option-name>
<wsimport-option-value>true</wsimport-option-value>
</wsimport-option>
- <wsimport-option>
<wsimport-option-name>wsdlLocation</wsimport-option-name>
<wsimport-option-value>http://ars-PC:8080/helloservice/HelloService?wsdl</wsimport-option-value>
</wsimport-option>
- <wsimport-option>
<wsimport-option-name>xnocompile</wsimport-option-name>
<wsimport-option-value>true</wsimport-option-value>
</wsimport-option>
- <wsimport-option>
<wsimport-option-name>xendorsed</wsimport-option-name>
<wsimport-option-value>true</wsimport-option-value>
</wsimport-option>
- <wsimport-option>
<wsimport-option-name>package</wsimport-option-name>
<wsimport-option-value>webclient</wsimport-option-value>
</wsimport-option>
</wsimport-options>
</client>
</clients>
</jax-ws>
1.2.3 jaxws-build.xml
<?xml version="1.0" encoding="UTF-8" ?>
- <!--
*** GENERATED FROM jax-ws.xml - DO NOT EDIT ! ***
*** TO MODIFY wsimport options USE Web Service node -> Edit WS Attributes ***
*** TO CHANGE TARGETS GENERATED TO jaxws-build.xml COPY THOSE ***
*** TARGETS TO ../build.xml AND MODIFY THAT FILE INSTEAD ***
-->
- <project xmlns:xalan="http://xml.apache.org/xslt" xmlns:webproject2="http://www.netbeans.org/ns/web-project/2" xmlns:jaxws="http://www.netbeans.org/ns/jax-ws/1">
- <!--
===================
JAX-WS WSGEN SECTION
===================
-->
- <!--
===================
JAX-WS WSIMPORT SECTION
===================
-->
- <target name="wsimport-init" depends="init">
<mkdir dir="${build.generated.sources.dir}/jax-ws" />
<property name="j2ee.platform.wsimport.classpath" value="${libs.jaxws21.classpath}" />
- <taskdef name="wsimport" classname="com.sun.tools.ws.ant.WsImport">
<classpath path="${java.home}/../lib/tools.jar:${j2ee.platform.wsimport.classpath}" />
</taskdef>
- <condition property="conf-dir" value="${conf.dir}/" else="">
<isset property="conf.dir" />
</condition>
</target>
- <target name="wsimport-client-HelloService" depends="wsimport-init">
<mkdir dir="${build.generated.dir}/jax-wsCache/HelloService" />
- <wsimport sourcedestdir="${build.generated.dir}/jax-wsCache/HelloService" destdir="${build.generated.dir}/jax-wsCache/HelloService" wsdl="${basedir}/${conf-dir}xml-resources/web-service-references/HelloService/wsdl/ars-PC_8080/helloservice/HelloService.wsdl" catalog="catalog.xml" extension="true" verbose="true" wsdlLocation="http://ars-PC:8080/helloservice/HelloService?wsdl" xnocompile="true" xendorsed="true" package="webclient">
<depends file="${basedir}/${conf-dir}xml-resources/web-service-references/HelloService/wsdl/ars-PC_8080/helloservice/HelloService.wsdl" />
<produces dir="${build.generated.dir}/jax-wsCache/HelloService" />
</wsimport>
- <copy todir="${build.generated.sources.dir}/jax-ws">
- <fileset dir="${build.generated.dir}/jax-wsCache/HelloService">
<include name="**/*.java" />
</fileset>
</copy>
</target>
- <target name="wsimport-client-clean-HelloService" depends="-init-project">
<delete dir="${build.generated.sources.dir}/jax-ws/webclient" />
<delete dir="${build.generated.dir}/jax-wsCache/HelloService" />
</target>
<target name="wsimport-client-generate" depends="wsimport-client-HelloService" />
</project>
1.3 The last change is the xml-resources folder created newly in the /src/conf/ folder as seen below:
Directory of C:\Users\ars\Desktop\webclientARS\src\conf\xml-resources\web-service-references\HelloService\wsdl\ars-PC_8080\helloservice
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:22 PM 2,593 HelloService.wsdl
03/11/2012 07:22 PM 1,024 HelloService.xsd_1.xsd
2 File(s) 3,617 bytes
The folder above contains the WSDL files.
1.4 webclient\web\WEB-INF\wsdl\ars-PC_8080\helloservice folder also has the wsdl files:
HelloService.wsdl and HelloService.xsd_1.xsd
2. The comparison of the output of the build phase to the WS-IMPORT phase.
2.1 As expected the build phase creates the dist folder which contains the distribution WAR. It also creates the web folder in the build folder.
Directory of C:\Users\ars\Desktop\webclientARS\build\web\WEB-INF
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM <DIR> classes
03/11/2012 08:18 PM 521 glassfish-web.xml
03/11/2012 08:18 PM 411 jax-ws-catalog.xml
03/11/2012 08:18 PM <DIR> wsdl
2 File(s) 932 bytes
...
webclient\build\web\WEB-INF\classes\webclient
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM 1,183 Hello.class
03/11/2012 08:18 PM 507 HelloResponse.class
03/11/2012 08:18 PM 2,691 HelloService.class
03/11/2012 08:18 PM 2,611 HelloServlet.class
03/11/2012 08:18 PM 490 Hello_Type.class
03/11/2012 08:18 PM 2,778 ObjectFactory.class
03/11/2012 08:18 PM 244 package-info.class
03/11/2012 08:18 PM 757 SayHello.class
03/11/2012 08:18 PM 863 SayHelloResponse.class
9 File(s) 12,124 bytes
...
webclient\build\web\WEB-INF\wsdl\ars-PC_8080\helloservice
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM 2,593 HelloService.wsdl
03/11/2012 08:18 PM 1,024 HelloService.xsd_1.xsd
2 File(s) 3,617 bytes
Nbproject folder shows some changes at genfiles.properties, which may be trivial.
2.2 It creates an empty ap-source-output folder in the build/generated-sources folder.
3. The comparison of the outcome of the execution phase to the build phase in terms of the files’ and folders’ of the project follows: Please notice the lengths of Hello.class and HelloServlet.class which reside at webclient\build\web\WEB-INF\classes\webclient
have increased after running the client.
An Analysis of JAX-WS usage of NetBeans2.1
Helloservice Content Comparison of development phases
A study of the differences in the contents of the folder and file structures of the four phases of helloservice shows us:
1. The changes in the nbproject folder that occur just after the creation of the webservice reflect the use of wsgen: Helloservice/nbproject/: The increase in the size of build-impl.xml , project.xml, genfiles.properties and the addition of jaxws-build.xml and jax-ws.xml
Please note the addition of helloservice\src\java\helloservice\endpoint\Hello.java to the src folder at the wsgen phase which can easily be observed at the netbeans IDE.
1.1 The addition to build-impl.xml is at the top:
<project xmlns:webproject1="http://www.netbeans.org/ns/web-project/1" xmlns:webproject2="http://www.netbeans.org/ns/web-project/2" xmlns:webproject3="http://www.netbeans.org/ns/web-project/3" basedir=".." default="default" name="helloserviceARS-impl">
<import file="jaxws-build.xml"/>
1.2 The addition to project.xml is to the extensions part:
<?xml version="1.0" encoding="UTF-8" ?>
- <project xmlns="http://www.netbeans.org/ns/project/1">
<type>org.netbeans.modules.web.project</type>
- <configuration>
- <buildExtensions xmlns="http://www.netbeans.org/ns/ant-build-extender/1">
<extension file="jaxws-build.xml" id="jaxws" />
</buildExtensions>
1.3 jax-ws.xml is:
<?xml version="1.0" encoding="UTF-8" ?>
- <jax-ws xmlns="http://www.netbeans.org/ns/jax-ws/1">
- <services>
- <service name="Hello">
<implementation-class>helloservice.endpoint.Hello</implementation-class>
</service>
</services>
<clients />
</jax-ws>
1.4 jaxws-build.xml is:
<?xml version="1.0" encoding="UTF-8" ?>
- <!--
*** GENERATED FROM jax-ws.xml - DO NOT EDIT ! ***
*** TO MODIFY wsimport options USE Web Service node -> Edit WS Attributes ***
*** TO CHANGE TARGETS GENERATED TO jaxws-build.xml COPY THOSE ***
*** TARGETS TO ../build.xml AND MODIFY THAT FILE INSTEAD ***
-->
- <project xmlns:xalan="http://xml.apache.org/xslt" xmlns:webproject2="http://www.netbeans.org/ns/web-project/2" xmlns:jaxws="http://www.netbeans.org/ns/jax-ws/1">
- <!--
===================
JAX-WS WSGEN SECTION
===================
-->
- <target name="wsgen-init" depends="init, -do-compile">
<mkdir dir="${build.generated.sources.dir}/jax-ws/resources/" />
<mkdir dir="${build.classes.dir}" />
<mkdir dir="${build.classes.dir}/META-INF" />
<property name="j2ee.platform.wsgen.classpath"value="${libs.jaxws21.classpath}" />
- <taskdef name="wsgen" classname="com.sun.tools.ws.ant.WsGen">
<classpath path="${java.home}/../lib/tools.jar:${build.classes.dir}:${j2ee.platform.wsgen.classpath}:${javac.classpath}" />
</taskdef>
</target>
- <target name="wsgen-Hello" depends="wsgen-init">
- <copy todir="${build.classes.dir}/META-INF">
<fileset dir="${webinf.dir}" includes="wsit-helloservice.endpoint.Hello.xml" />
</copy>
- <wsgen sourcedestdir="${build.generated.sources.dir}/jax-ws" resourcedestdir="${build.generated.sources.dir}/jax-ws/resources/" destdir="${build.generated.sources.dir}/jax-ws" verbose="true" xendorsed="true" keep="true" genwsdl="true" sei="helloservice.endpoint.Hello">
<classpath path="${java.home}/../lib/tools.jar:${build.classes.dir}:${j2ee.platform.wsgen.classpath}:${javac.classpath}" />
</wsgen>
</target>
- <!--
===================
JAX-WS WSIMPORT SECTION
===================
-->
</project>
1.5 The changes in Helloservice/nbproject/genfiles.properties : are related with CRC values:
nbproject/build-impl.xml.data.CRC32=eb7dd51f
nbproject/build-impl.xml.script.CRC32=d96d99ca
nbproject/build-impl.xml.stylesheet.CRC32=8ca9f0e6@1.31.1.1
nbproject/jaxws-build.xml.stylesheet.CRC32=03b77b15
Please note that a new CRC record is created for jaxws-build.xml
2. After we build the helloservice project: the helloservice/dist and helloservice/build folders appear.
The build folder has:
03/10/2012 11:25 PM <DIR> empty
03/10/2012 11:25 PM <DIR> generated-sources
03/10/2012 11:25 PM <DIR> web
\build\empty is empty.
\build\generated-sources\ap-source-output is empty.
\build\web has the compile-build outputs of the index.jsp and the Hello.java
03/10/2012 11:25 PM 369 index.jsp
03/10/2012 11:25 PM <DIR> META-INF
03/10/2012 11:25 PM <DIR> WEB-INF
Directory of C:\Users\ars\Desktop\helloserviceARS3build\build\web\META-INF
03/10/2012 11:25 PM 25 MANIFEST.MF
Directory of C:\Users\ars\Desktop\helloserviceARS3build\build\web\WEB-INF
03/10/2012 11:25 PM <DIR> classes
03/10/2012 11:25 PM 524 glassfish-web.xml
03/10/2012 11:25 PM 405 web.xml
\build\web\WEB-INF\classes\helloservice\endpoint\Hello.class
3. After we run the helloservice project and create the webservice on the server:
There is a trivial change in the nbproject/private/private.xml file: The open-files item is added.
<?xml version="1.0" encoding="UTF-8"?>
<project-private xmlns="http://www.netbeans.org/ns/project-private/1">
<editor-bookmarks xmlns="http://www.netbeans.org/ns/editor-bookmarks/1"/>
<open-files xmlns="http://www.netbeans.org/ns/projectui-open-files/1">
<file>file:/C:/Users/ars/Desktop/helloserviceARS/web/index.jsp</file>
</open-files>
</project-private>
A study of the differences in the contents of the folder and file structures of the four phases of helloservice shows us:
1. The changes in the nbproject folder that occur just after the creation of the webservice reflect the use of wsgen: Helloservice/nbproject/: The increase in the size of build-impl.xml , project.xml, genfiles.properties and the addition of jaxws-build.xml and jax-ws.xml
Please note the addition of helloservice\src\java\helloservice\endpoint\Hello.java to the src folder at the wsgen phase which can easily be observed at the netbeans IDE.
1.1 The addition to build-impl.xml is at the top:
<project xmlns:webproject1="http://www.netbeans.org/ns/web-project/1" xmlns:webproject2="http://www.netbeans.org/ns/web-project/2" xmlns:webproject3="http://www.netbeans.org/ns/web-project/3" basedir=".." default="default" name="helloserviceARS-impl">
<import file="jaxws-build.xml"/>
1.2 The addition to project.xml is to the extensions part:
<?xml version="1.0" encoding="UTF-8" ?>
- <project xmlns="http://www.netbeans.org/ns/project/1">
<type>org.netbeans.modules.web.project</type>
- <configuration>
- <buildExtensions xmlns="http://www.netbeans.org/ns/ant-build-extender/1">
<extension file="jaxws-build.xml" id="jaxws" />
</buildExtensions>
1.3 jax-ws.xml is:
<?xml version="1.0" encoding="UTF-8" ?>
- <jax-ws xmlns="http://www.netbeans.org/ns/jax-ws/1">
- <services>
- <service name="Hello">
<implementation-class>helloservice.endpoint.Hello</implementation-class>
</service>
</services>
<clients />
</jax-ws>
1.4 jaxws-build.xml is:
<?xml version="1.0" encoding="UTF-8" ?>
- <!--
*** GENERATED FROM jax-ws.xml - DO NOT EDIT ! ***
*** TO MODIFY wsimport options USE Web Service node -> Edit WS Attributes ***
*** TO CHANGE TARGETS GENERATED TO jaxws-build.xml COPY THOSE ***
*** TARGETS TO ../build.xml AND MODIFY THAT FILE INSTEAD ***
-->
- <project xmlns:xalan="http://xml.apache.org/xslt" xmlns:webproject2="http://www.netbeans.org/ns/web-project/2" xmlns:jaxws="http://www.netbeans.org/ns/jax-ws/1">
- <!--
===================
JAX-WS WSGEN SECTION
===================
-->
- <target name="wsgen-init" depends="init, -do-compile">
<mkdir dir="${build.generated.sources.dir}/jax-ws/resources/" />
<mkdir dir="${build.classes.dir}" />
<mkdir dir="${build.classes.dir}/META-INF" />
<property name="j2ee.platform.wsgen.classpath"value="${libs.jaxws21.classpath}" />
- <taskdef name="wsgen" classname="com.sun.tools.ws.ant.WsGen">
<classpath path="${java.home}/../lib/tools.jar:${build.classes.dir}:${j2ee.platform.wsgen.classpath}:${javac.classpath}" />
</taskdef>
</target>
- <target name="wsgen-Hello" depends="wsgen-init">
- <copy todir="${build.classes.dir}/META-INF">
<fileset dir="${webinf.dir}" includes="wsit-helloservice.endpoint.Hello.xml" />
</copy>
- <wsgen sourcedestdir="${build.generated.sources.dir}/jax-ws" resourcedestdir="${build.generated.sources.dir}/jax-ws/resources/" destdir="${build.generated.sources.dir}/jax-ws" verbose="true" xendorsed="true" keep="true" genwsdl="true" sei="helloservice.endpoint.Hello">
<classpath path="${java.home}/../lib/tools.jar:${build.classes.dir}:${j2ee.platform.wsgen.classpath}:${javac.classpath}" />
</wsgen>
</target>
- <!--
===================
JAX-WS WSIMPORT SECTION
===================
-->
</project>
1.5 The changes in Helloservice/nbproject/genfiles.properties : are related with CRC values:
nbproject/build-impl.xml.data.CRC32=eb7dd51f
nbproject/build-impl.xml.script.CRC32=d96d99ca
nbproject/build-impl.xml.stylesheet.CRC32=8ca9f0e6@1.31.1.1
nbproject/jaxws-build.xml.stylesheet.CRC32=03b77b15
Please note that a new CRC record is created for jaxws-build.xml
2. After we build the helloservice project: the helloservice/dist and helloservice/build folders appear.
The build folder has:
03/10/2012 11:25 PM <DIR> empty
03/10/2012 11:25 PM <DIR> generated-sources
03/10/2012 11:25 PM <DIR> web
\build\empty is empty.
\build\generated-sources\ap-source-output is empty.
\build\web has the compile-build outputs of the index.jsp and the Hello.java
03/10/2012 11:25 PM 369 index.jsp
03/10/2012 11:25 PM <DIR> META-INF
03/10/2012 11:25 PM <DIR> WEB-INF
Directory of C:\Users\ars\Desktop\helloserviceARS3build\build\web\META-INF
03/10/2012 11:25 PM 25 MANIFEST.MF
Directory of C:\Users\ars\Desktop\helloserviceARS3build\build\web\WEB-INF
03/10/2012 11:25 PM <DIR> classes
03/10/2012 11:25 PM 524 glassfish-web.xml
03/10/2012 11:25 PM 405 web.xml
\build\web\WEB-INF\classes\helloservice\endpoint\Hello.class
3. After we run the helloservice project and create the webservice on the server:
There is a trivial change in the nbproject/private/private.xml file: The open-files item is added.
<?xml version="1.0" encoding="UTF-8"?>
<project-private xmlns="http://www.netbeans.org/ns/project-private/1">
<editor-bookmarks xmlns="http://www.netbeans.org/ns/editor-bookmarks/1"/>
<open-files xmlns="http://www.netbeans.org/ns/projectui-open-files/1">
<file>file:/C:/Users/ars/Desktop/helloserviceARS/web/index.jsp</file>
</open-files>
</project-private>
Tuesday 13 March 2012
An Analysis of JAX-WS usage of NetBeans 1.2.2 (Anaysis of the webclient)
An Analysis of JAX-WS usage of NetBeans 1.2.2 (Anaysis of the webclient)
I will compare the files and folders created at the 4 phases of webclient here.
1. The initial phase. This is the beginning condition of the project without any WS import.
The project window can be seen at "An Analysis of JAX-WS usage of NetBeans 1.2.1 (Production of the webclient)" number 1.
The wsimport phase is realized in "An Analysis of JAX-WS usage of NetBeans 1.2.1 (Production of the webclient)" number 2.
The wsimport process is realized at number 3. Picture 3.5 shows the final status of the project window after the wsimport.
You can find the comparison of recursive dir outputs between the initial and post-wsimport status of the project.
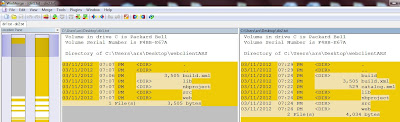
As seen above, a new build folder is created although we have not done a project build operation. Also a catalog.xml file is created.
Directory of C:\Users\ars\Desktop\webclientARS\build
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> generated
03/11/2012 07:24 PM <DIR> generated-sources
0 File(s) 0 bytes
As seen above two new folders named generated and generated-sources are created in the new build folder. These folders contain the generated java files related with the webservice. These files have to be transmitted as XML files and converted via JAXB to generate the java sources but to prove this we will have to wait till the second phase of our small researh when we wil study the contents of the files psecially build xmls.
Directory of C:\Users\ars\Desktop\webclientARS\build\generated
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> jax-wsCache
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\generated\jax-wsCache
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> HelloService
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\generated\jax-wsCache\HelloService
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> webclient
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\generated\jax-wsCache\HelloService\webclient
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:22 PM 1,751 Hello.java
03/11/2012 07:22 PM 745 HelloResponse.java
03/11/2012 07:22 PM 2,957 HelloService.java
03/11/2012 07:22 PM 718 Hello_Type.java
03/11/2012 07:22 PM 3,693 ObjectFactory.java
03/11/2012 07:22 PM 103 package-info.java
03/11/2012 07:22 PM 1,347 SayHello.java
03/11/2012 07:22 PM 1,481 SayHelloResponse.java
8 File(s) 12,795 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\generated-sources
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> jax-ws
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\generated-sources\jax-ws
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> webclient
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\generated-sources\jax-ws\webclient
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:22 PM 1,751 Hello.java
03/11/2012 07:22 PM 745 HelloResponse.java
03/11/2012 07:22 PM 2,957 HelloService.java
03/11/2012 07:22 PM 718 Hello_Type.java
03/11/2012 07:22 PM 3,693 ObjectFactory.java
03/11/2012 07:22 PM 103 package-info.java
03/11/2012 07:22 PM 1,347 SayHello.java
03/11/2012 07:22 PM 1,481 SayHelloResponse.java
8 File(s) 12,795 bytes
Also, the nbproject folder has changed similar to the webservice creation phase. There is a new Jaxws-build.xml and jax-ws.xml addition.
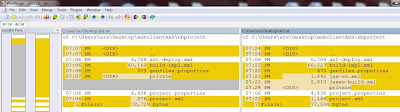
The last change is xml-resources folder created newly in the /src/conf/ folder as seen below:
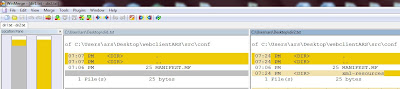
Directory of C:\Users\ars\Desktop\webclientARS\src\conf\xml-resources
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> web-service-references
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\src\conf\xml-resources\web-service-references
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> HelloService
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\src\conf\xml-resources\web-service-references\HelloService
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> wsdl
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\src\conf\xml-resources\web-service-references\HelloService\wsdl
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> ars-PC_8080
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\src\conf\xml-resources\web-service-references\HelloService\wsdl\ars-PC_8080
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> helloservice
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\src\conf\xml-resources\web-service-references\HelloService\wsdl\ars-PC_8080\helloservice
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:22 PM 2,593 HelloService.wsdl
03/11/2012 07:22 PM 1,024 HelloService.xsd_1.xsd
2 File(s) 3,617 bytes
The folder above contains the WSDL files.

Also the WEB-INF folder contains the WSDL files related to the ars-PC_8080/helloservice/Hello folders.
2. The comparison of the output of the build phase to the WS-IMPORT phase. As expected the build phase creates the dist folder which contains the distribution WAR. It also creates the web folder in the build folder.
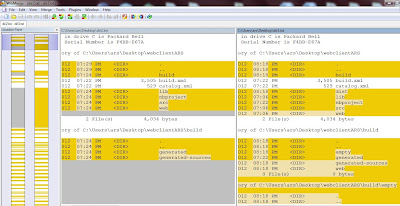
It creates an empty ap-source-output folder in the /build/generated-sources folder.
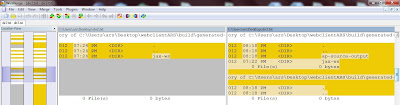
Newly created:
Directory of C:\Users\ars\Desktop\webclientARS\build\web
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM 368 index.jsp
03/11/2012 08:18 PM <DIR> META-INF
03/11/2012 08:18 PM <DIR> WEB-INF
1 File(s) 368 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\web\META-INF
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM 25 MANIFEST.MF
1 File(s) 25 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\web\WEB-INF
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM <DIR> classes
03/11/2012 08:18 PM 521 glassfish-web.xml
03/11/2012 08:18 PM 411 jax-ws-catalog.xml
03/11/2012 08:18 PM <DIR> wsdl
2 File(s) 932 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\web\WEB-INF\classes
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM <DIR> webclient
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\web\WEB-INF\classes\webclient
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM 1,183 Hello.class
03/11/2012 08:18 PM 507 HelloResponse.class
03/11/2012 08:18 PM 2,691 HelloService.class
03/11/2012 08:18 PM 2,611 HelloServlet.class
03/11/2012 08:18 PM 490 Hello_Type.class
03/11/2012 08:18 PM 2,778 ObjectFactory.class
03/11/2012 08:18 PM 244 package-info.class
03/11/2012 08:18 PM 757 SayHello.class
03/11/2012 08:18 PM 863 SayHelloResponse.class
9 File(s) 12,124 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\web\WEB-INF\wsdl
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM <DIR> ars-PC_8080
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\web\WEB-INF\wsdl\ars-PC_8080
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM <DIR> helloservice
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\web\WEB-INF\wsdl\ars-PC_8080\helloservice
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM 2,593 HelloService.wsdl
03/11/2012 08:18 PM 1,024 HelloService.xsd_1.xsd
2 File(s) 3,617 bytes
Directory of C:\Users\ars\Desktop\webclientARS\dist
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM 20,287 webclientARS.war
1 File(s) 20,287 bytes
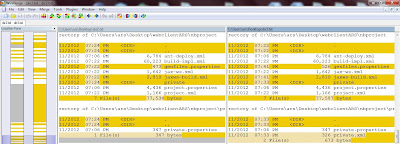
Nbproject folder shows some changes at genfiles.properties, which may be trivial but we will see.
3. The comparison of the outcome of the execution phase to the build phase in terms of the files’ and folders’ of the project follows:
The execution output at the NetBeans IDE:
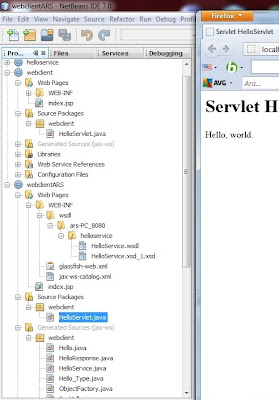
The only change can be observed at the classes folder. Two new files have been created .netbeans_automatic_build and .netbeans_update_resources of length 0 each.
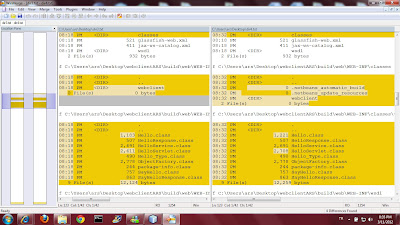
Please notice the lengths of Hello.class and HelloServlet.class have increased.
I will compare the files and folders created at the 4 phases of webclient here.
1. The initial phase. This is the beginning condition of the project without any WS import.
The project window can be seen at "An Analysis of JAX-WS usage of NetBeans 1.2.1 (Production of the webclient)" number 1.
The wsimport phase is realized in "An Analysis of JAX-WS usage of NetBeans 1.2.1 (Production of the webclient)" number 2.
The wsimport process is realized at number 3. Picture 3.5 shows the final status of the project window after the wsimport.
You can find the comparison of recursive dir outputs between the initial and post-wsimport status of the project.
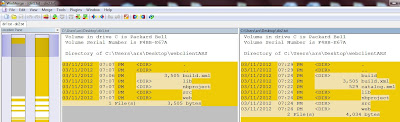
As seen above, a new build folder is created although we have not done a project build operation. Also a catalog.xml file is created.
Directory of C:\Users\ars\Desktop\webclientARS\build
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> generated
03/11/2012 07:24 PM <DIR> generated-sources
0 File(s) 0 bytes
As seen above two new folders named generated and generated-sources are created in the new build folder. These folders contain the generated java files related with the webservice. These files have to be transmitted as XML files and converted via JAXB to generate the java sources but to prove this we will have to wait till the second phase of our small researh when we wil study the contents of the files psecially build xmls.
Directory of C:\Users\ars\Desktop\webclientARS\build\generated
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> jax-wsCache
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\generated\jax-wsCache
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> HelloService
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\generated\jax-wsCache\HelloService
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> webclient
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\generated\jax-wsCache\HelloService\webclient
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:22 PM 1,751 Hello.java
03/11/2012 07:22 PM 745 HelloResponse.java
03/11/2012 07:22 PM 2,957 HelloService.java
03/11/2012 07:22 PM 718 Hello_Type.java
03/11/2012 07:22 PM 3,693 ObjectFactory.java
03/11/2012 07:22 PM 103 package-info.java
03/11/2012 07:22 PM 1,347 SayHello.java
03/11/2012 07:22 PM 1,481 SayHelloResponse.java
8 File(s) 12,795 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\generated-sources
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> jax-ws
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\generated-sources\jax-ws
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> webclient
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\generated-sources\jax-ws\webclient
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:22 PM 1,751 Hello.java
03/11/2012 07:22 PM 745 HelloResponse.java
03/11/2012 07:22 PM 2,957 HelloService.java
03/11/2012 07:22 PM 718 Hello_Type.java
03/11/2012 07:22 PM 3,693 ObjectFactory.java
03/11/2012 07:22 PM 103 package-info.java
03/11/2012 07:22 PM 1,347 SayHello.java
03/11/2012 07:22 PM 1,481 SayHelloResponse.java
8 File(s) 12,795 bytes
Also, the nbproject folder has changed similar to the webservice creation phase. There is a new Jaxws-build.xml and jax-ws.xml addition.
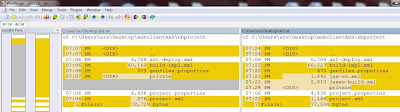
The last change is xml-resources folder created newly in the /src/conf/ folder as seen below:
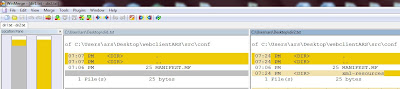
Directory of C:\Users\ars\Desktop\webclientARS\src\conf\xml-resources
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> web-service-references
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\src\conf\xml-resources\web-service-references
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> HelloService
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\src\conf\xml-resources\web-service-references\HelloService
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> wsdl
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\src\conf\xml-resources\web-service-references\HelloService\wsdl
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> ars-PC_8080
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\src\conf\xml-resources\web-service-references\HelloService\wsdl\ars-PC_8080
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:24 PM <DIR> helloservice
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\src\conf\xml-resources\web-service-references\HelloService\wsdl\ars-PC_8080\helloservice
03/11/2012 07:24 PM <DIR> .
03/11/2012 07:24 PM <DIR> ..
03/11/2012 07:22 PM 2,593 HelloService.wsdl
03/11/2012 07:22 PM 1,024 HelloService.xsd_1.xsd
2 File(s) 3,617 bytes
The folder above contains the WSDL files.

Also the WEB-INF folder contains the WSDL files related to the ars-PC_8080/helloservice/Hello folders.
2. The comparison of the output of the build phase to the WS-IMPORT phase. As expected the build phase creates the dist folder which contains the distribution WAR. It also creates the web folder in the build folder.
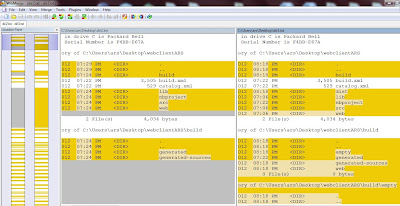
It creates an empty ap-source-output folder in the /build/generated-sources folder.
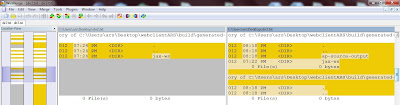
Newly created:
Directory of C:\Users\ars\Desktop\webclientARS\build\web
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM 368 index.jsp
03/11/2012 08:18 PM <DIR> META-INF
03/11/2012 08:18 PM <DIR> WEB-INF
1 File(s) 368 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\web\META-INF
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM 25 MANIFEST.MF
1 File(s) 25 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\web\WEB-INF
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM <DIR> classes
03/11/2012 08:18 PM 521 glassfish-web.xml
03/11/2012 08:18 PM 411 jax-ws-catalog.xml
03/11/2012 08:18 PM <DIR> wsdl
2 File(s) 932 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\web\WEB-INF\classes
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM <DIR> webclient
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\web\WEB-INF\classes\webclient
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM 1,183 Hello.class
03/11/2012 08:18 PM 507 HelloResponse.class
03/11/2012 08:18 PM 2,691 HelloService.class
03/11/2012 08:18 PM 2,611 HelloServlet.class
03/11/2012 08:18 PM 490 Hello_Type.class
03/11/2012 08:18 PM 2,778 ObjectFactory.class
03/11/2012 08:18 PM 244 package-info.class
03/11/2012 08:18 PM 757 SayHello.class
03/11/2012 08:18 PM 863 SayHelloResponse.class
9 File(s) 12,124 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\web\WEB-INF\wsdl
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM <DIR> ars-PC_8080
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\web\WEB-INF\wsdl\ars-PC_8080
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM <DIR> helloservice
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\webclientARS\build\web\WEB-INF\wsdl\ars-PC_8080\helloservice
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM 2,593 HelloService.wsdl
03/11/2012 08:18 PM 1,024 HelloService.xsd_1.xsd
2 File(s) 3,617 bytes
Directory of C:\Users\ars\Desktop\webclientARS\dist
03/11/2012 08:18 PM <DIR> .
03/11/2012 08:18 PM <DIR> ..
03/11/2012 08:18 PM 20,287 webclientARS.war
1 File(s) 20,287 bytes
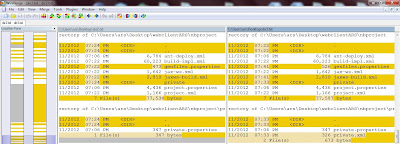
Nbproject folder shows some changes at genfiles.properties, which may be trivial but we will see.
3. The comparison of the outcome of the execution phase to the build phase in terms of the files’ and folders’ of the project follows:
The execution output at the NetBeans IDE:
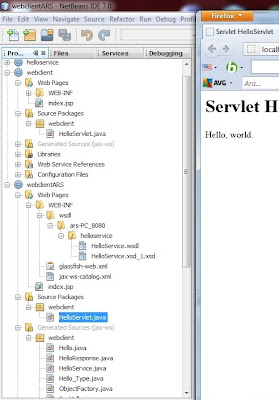
The only change can be observed at the classes folder. Two new files have been created .netbeans_automatic_build and .netbeans_update_resources of length 0 each.
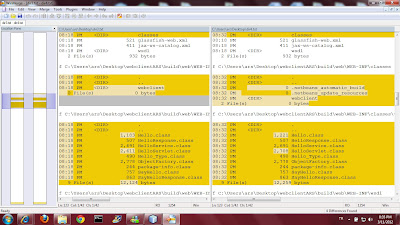
Please notice the lengths of Hello.class and HelloServlet.class have increased.
Monday 12 March 2012
An Analysis of JAX-WS usage of NetBeans 1.2.1 (Production of the webclient)
This is a closer look at JAX-WS examples provided by JAVAEE5/JAVAEE6 tutorials. The names of the examples are helloservice, webclient and appclient. This is the continuation of the first part. I will explain the development of the webclient first in
An Analysis of JAX-WS usage of NetBeans 1.2.1 (Production of the webclient)
And then I will compare dynamically the changes in the directory and project levels at the different phases of the project in the succeeding section
An Analysis of JAX-WS usage of NetBeans 1.2.2 (Anaysis of the webclient)
1 webclient:
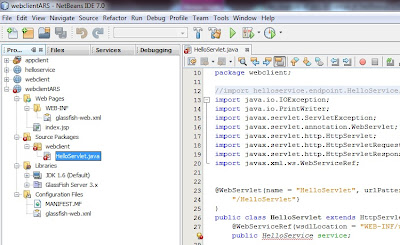
Please notice the configuration files and other folders, there is nothing related to the webservic accept the WSDL address we entered into HelloServlet.java:
HelloServlet.java
/*
* Copyright 2011 Oracle and/or its affiliates.
* All rights reserved. You may not modify, use,
* reproduce, or distribute this software except in
* compliance with the terms of the License at:
* http://developers.sun.com/license/berkeley_license.html
*/
package webclient;
//import helloservice.endpoint.HelloService;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.xml.ws.WebServiceRef;
@WebServlet(name = "HelloServlet", urlPatterns = {
"/HelloServlet"}
)
public class HelloServlet extends HttpServlet {
@WebServiceRef(wsdlLocation = "WEB-INF/wsdl/ars-PC_8080/helloservice/HelloService.wsdl")
public HelloService service;
/**
* Processes requests for both HTTP <code>GET</code>
* and <code>POST</code> methods.
* @param request servlet request
* @param response servlet response
* @throws ServletException if a servlet-specific error occurs
* @throws IOException if an I/O error occurs
*/
protected void processRequest(
HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html;charset=UTF-8");
PrintWriter out = response.getWriter();
try {
out.println("<html lang=\"en\">");
out.println("<head>");
out.println("<title>Servlet HelloServlet</title>");
out.println("</head>");
out.println("<body>");
out.println(
"<h1>Servlet HelloServlet at " + request.getContextPath()
+ "</h1>");
out.println("<p>" + sayHello("world") + "</p>");
out.println("</body>");
out.println("</html>");
} finally {
out.close();
}
}
// <editor-fold defaultstate="collapsed" desc="HttpServlet methods. Click on the + sign on the left to edit the code.">
/**
* Handles the HTTP <code>GET</code> method.
* @param request servlet request
* @param response servlet response
* @throws ServletException if a servlet-specific error occurs
* @throws IOException if an I/O error occurs
*/
@Override
protected void doGet(
HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
processRequest(request, response);
}
/**
* Handles the HTTP <code>POST</code> method.
* @param request servlet request
* @param response servlet response
* @throws ServletException if a servlet-specific error occurs
* @throws IOException if an I/O error occurs
*/
@Override
protected void doPost(
HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
processRequest(request, response);
}
/**
* Returns a short description of the servlet.
* @return a String containing servlet description
*/
@Override
public String getServletInfo() {
return "Short description";
} // </editor-fold>
private String sayHello(java.lang.String arg0) {
//helloservice.endpoint.Hello port = service.getHelloPort();
webclient.Hello port = service.getHelloPort();
return port.sayHello(arg0);
}
}
2 The WSDL adress given above as:
@WebServiceRef(wsdlLocation = "WEB-INF/wsdl/ars-PC_8080/helloservice/HelloService.wsdl")
Can be found by doing:
2.1 Open the GlassFish server Admin
2.2 Go to applications, helloservice and to the bottom of the page you see:
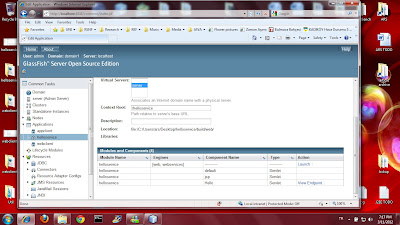
2.3 Click on the View Endpoint at the right bottom corner:
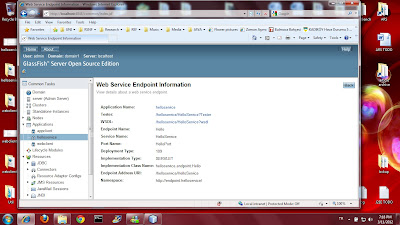
2.4 Click on the highlighted line which ends with wsdl. Here is the wsdl address:
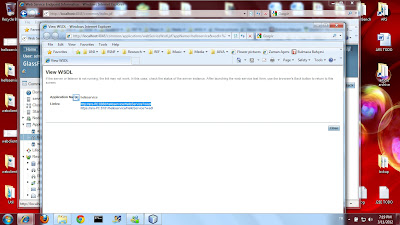
3. After we get the WSDL adress for the webservice we had created with the helloservice project we can set up for the wsimport of this service.
3.1 Right click on webclient and select new, select Web Service Client:
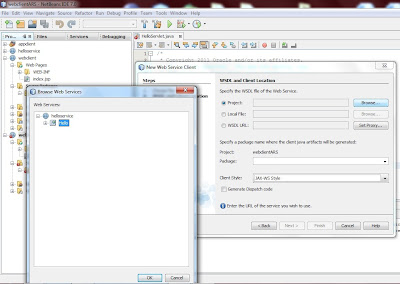
3.2 Enter the WSDL adress that you have taken from the GlassFish Admin. Select the package your imported and generated java sources will reside.
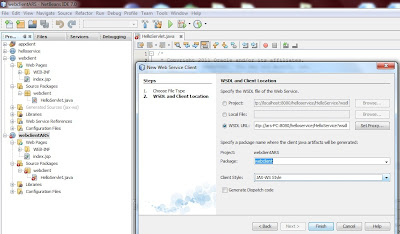
3.3 When you select finish it begins to parse:

3.4 The output window shows the generated code:
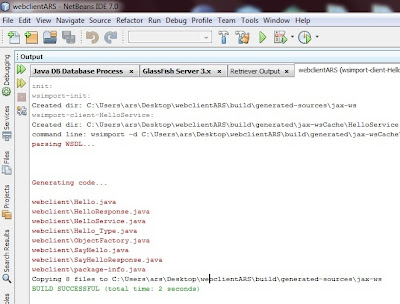
3.5 The project window becomes as shown below. At this point we can pursue our goal of dynamically comparing the changes in the directory and project levels at the different phases of the project:
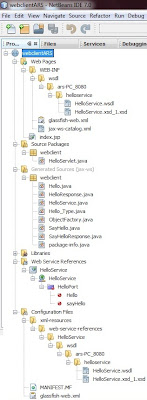
1.2.2 Please proceed to the section
An Analysis of JAX-WS usage of NetBeans 1.2.2 (Analysis of the webclient)
An Analysis of JAX-WS usage of NetBeans 1.2.1 (Production of the webclient)
And then I will compare dynamically the changes in the directory and project levels at the different phases of the project in the succeeding section
An Analysis of JAX-WS usage of NetBeans 1.2.2 (Anaysis of the webclient)
1 webclient:
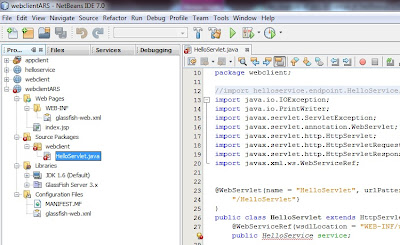
Please notice the configuration files and other folders, there is nothing related to the webservic accept the WSDL address we entered into HelloServlet.java:
HelloServlet.java
/*
* Copyright 2011 Oracle and/or its affiliates.
* All rights reserved. You may not modify, use,
* reproduce, or distribute this software except in
* compliance with the terms of the License at:
* http://developers.sun.com/license/berkeley_license.html
*/
package webclient;
//import helloservice.endpoint.HelloService;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.xml.ws.WebServiceRef;
@WebServlet(name = "HelloServlet", urlPatterns = {
"/HelloServlet"}
)
public class HelloServlet extends HttpServlet {
@WebServiceRef(wsdlLocation = "WEB-INF/wsdl/ars-PC_8080/helloservice/HelloService.wsdl")
public HelloService service;
/**
* Processes requests for both HTTP <code>GET</code>
* and <code>POST</code> methods.
* @param request servlet request
* @param response servlet response
* @throws ServletException if a servlet-specific error occurs
* @throws IOException if an I/O error occurs
*/
protected void processRequest(
HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html;charset=UTF-8");
PrintWriter out = response.getWriter();
try {
out.println("<html lang=\"en\">");
out.println("<head>");
out.println("<title>Servlet HelloServlet</title>");
out.println("</head>");
out.println("<body>");
out.println(
"<h1>Servlet HelloServlet at " + request.getContextPath()
+ "</h1>");
out.println("<p>" + sayHello("world") + "</p>");
out.println("</body>");
out.println("</html>");
} finally {
out.close();
}
}
// <editor-fold defaultstate="collapsed" desc="HttpServlet methods. Click on the + sign on the left to edit the code.">
/**
* Handles the HTTP <code>GET</code> method.
* @param request servlet request
* @param response servlet response
* @throws ServletException if a servlet-specific error occurs
* @throws IOException if an I/O error occurs
*/
@Override
protected void doGet(
HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
processRequest(request, response);
}
/**
* Handles the HTTP <code>POST</code> method.
* @param request servlet request
* @param response servlet response
* @throws ServletException if a servlet-specific error occurs
* @throws IOException if an I/O error occurs
*/
@Override
protected void doPost(
HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
processRequest(request, response);
}
/**
* Returns a short description of the servlet.
* @return a String containing servlet description
*/
@Override
public String getServletInfo() {
return "Short description";
} // </editor-fold>
private String sayHello(java.lang.String arg0) {
//helloservice.endpoint.Hello port = service.getHelloPort();
webclient.Hello port = service.getHelloPort();
return port.sayHello(arg0);
}
}
2 The WSDL adress given above as:
@WebServiceRef(wsdlLocation = "WEB-INF/wsdl/ars-PC_8080/helloservice/HelloService.wsdl")
Can be found by doing:
2.1 Open the GlassFish server Admin
2.2 Go to applications, helloservice and to the bottom of the page you see:
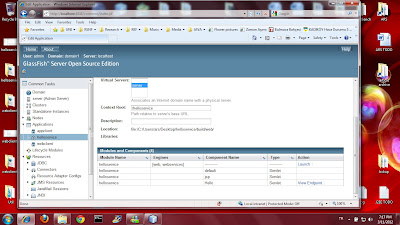
2.3 Click on the View Endpoint at the right bottom corner:
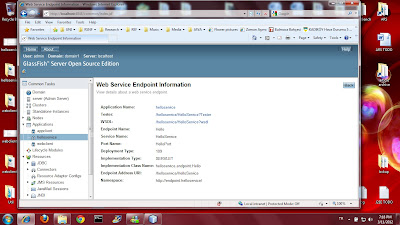
2.4 Click on the highlighted line which ends with wsdl. Here is the wsdl address:
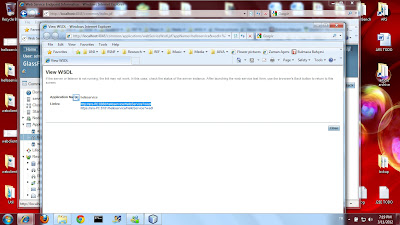
3. After we get the WSDL adress for the webservice we had created with the helloservice project we can set up for the wsimport of this service.
3.1 Right click on webclient and select new, select Web Service Client:
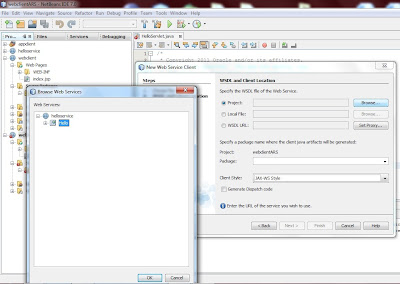
3.2 Enter the WSDL adress that you have taken from the GlassFish Admin. Select the package your imported and generated java sources will reside.
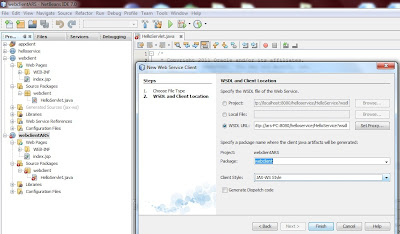
3.3 When you select finish it begins to parse:

3.4 The output window shows the generated code:
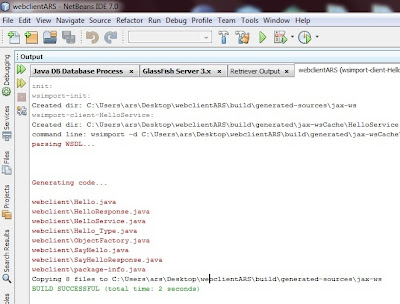
3.5 The project window becomes as shown below. At this point we can pursue our goal of dynamically comparing the changes in the directory and project levels at the different phases of the project:
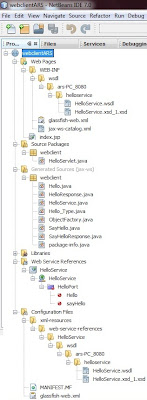
1.2.2 Please proceed to the section
An Analysis of JAX-WS usage of NetBeans 1.2.2 (Analysis of the webclient)
Sunday 11 March 2012
An Analysis of JAX-WS usage of NetBeans 1.1
This is a closer look at JAX-WS examples provided by JAVAEE5/JAVAEE6 tutorials. The names of the examples are helloservice, webclient and appclient.
First, I will provide a dynamic analysis of the file structures and their changes through four phases: init, wsgen/wsimport, build and run.
The dynamic analysis will provide pictures from NetBeans own project window and also external recursive dir listings accompanied by sources. The dir listings will be compared with WinMerge and produced outcomes will be used to compare the files in the different stages of the projects with WinMerge again.
Second, the outcomes of the comparisons will be used as clues for further analysis of build xml files namely the contents of the files, to better understand and pinpoint the jax-ws mechanism used by netbeans.
There will be plenty of pictures and WinMerge outputs to make this a little bit more humane.
1.1 Helloservice
1.1.1 Helloservice(init) initially it does not have a service yet. Helloservice.endpoint will be the location of the webservice.

1.1.2 HelloService’s create the service source phase includes creating the service itself using NetBeans’s New-->WebService command. After this project window looks like this:
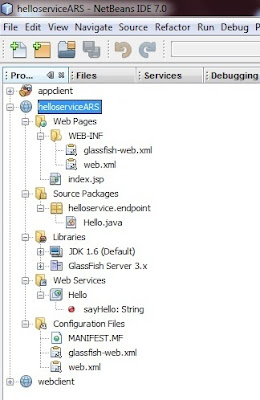
The service Hello.java is created. We write into Hello.java:
package helloservice.endpoint;
import javax.jws.WebService;
import javax.jws.WebMethod;
@WebService
public class Hello {
private String message = new String("Hello, ");
public void Hello() {
}
@WebMethod
public String sayHello(String name) {
return message + name + ".";
}
}
The result of recursive dir comparisons with WinMerge showschanges basicly in the
helloserviceARS\src\java\helloservice library which complies with the NetBeans project window picture above but also shows changes in the
helloserviceARS\nbproject
directory which is not displayed in the project window. The WinMerge output follows:

As can be observed above, the size of the build-impl.xml file has increased something like a single line and a new file named jaxws-build.xml is added along with jaxws.xml.
Here jax-ws appears to exist in the initial phase due to a delinquency where I first created the service and then deleted it to collect data but forget that an empty jax-ws.xml remains behind. Do not worry the second stage of this report will handle and explain all the irregularities that may happen at this stage. The second phase will explain based on the contents of files and verify the outcome of the first phase.
1.1.3 When we build the helloservice project, the project window looks like this:
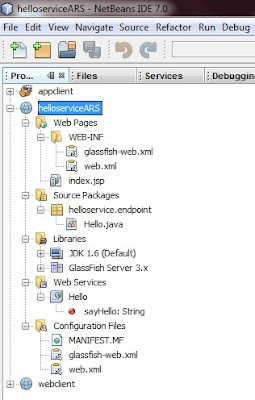
A comparison of the project directories after and before build shows that a directory named build is created and also :
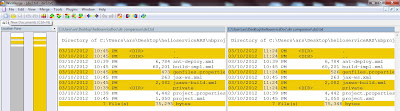
Helloservice/nbproject/genfiles.properties has been altered.
The newly created build directory contains:
Directory of C:\Users\ars\Desktop\helloserviceARS\build
03/10/2012 11:25 PM <DIR> .
03/10/2012 11:25 PM <DIR> ..
03/10/2012 11:25 PM <DIR> empty
03/10/2012 11:25 PM <DIR> generated-sources
03/10/2012 11:25 PM <DIR> web
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\helloserviceARS\build\empty
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\helloserviceARS\build\generated-sources
03/10/2012 11:25 PM <DIR> ap-source-output
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\helloserviceARS\build\generated-sources\ap-source-output
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\helloserviceARS\build\web
03/10/2012 11:25 PM 369 index.jsp
03/10/2012 11:25 PM <DIR> META-INF
03/10/2012 11:25 PM <DIR> WEB-INF
1 File(s) 369 bytes
Directory of C:\Users\ars\Desktop\helloserviceARS\build\web\META-INF
03/10/2012 11:25 PM 25 MANIFEST.MF
1 File(s) 25 bytes
Directory of C:\Users\ars\Desktop\helloserviceARS\build\web\WEB-INF
03/10/2012 11:25 PM <DIR> classes
03/10/2012 11:25 PM 524 glassfish-web.xml
03/10/2012 11:25 PM 405 web.xml
2 File(s) 929 bytes
Directory of C:\Users\ars\Desktop\helloserviceARS\build\web\WEB-INF\classes
03/10/2012 11:25 PM <DIR> helloservice
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\helloserviceARS\build\web\WEB-INF\classes\helloservice
03/10/2012 11:25 PM <DIR> endpoint
0 File(s) 0 bytes
Directory of C:\Users\ars\Desktop\helloserviceARS\build\web\WEB-INF\classes\helloservice\endpoint
03/10/2012 11:25 PM 889 Hello.class
1 File(s) 889 bytes
Directory of C:\Users\ars\Desktop\helloserviceARS\dist
03/10/2012 11:25 PM 3,519 helloserviceARS.war
1 File(s) 3,519 bytes
1.1.4 When we run the project the outcome at the project window is this:
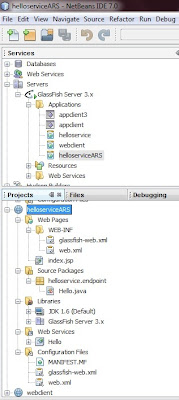
I used the project name helloserviceARS not to delete and remake helloservice. As seen above, helloserviceARS is created as an application on the Glassfish server. The project window does not show any differences but the recursive dir comparison:

Shows a minor change in the helloservice/nbproject/private/private.xml file.
Thursday 8 March 2012
JAVAEE5-JAXB solution examples2
The Java(TM) Web Services Tutorial at http://java.sun.com/webservices/docs/1.4/tutorial/doc/JAXBUsing.html has a few JAXB examples some of which still be made to work. These examples are similar to JAVAEE5-JAXB examples. I will provide the working solution for one of these examples which can also be used to make the other examples at JAVAEE5 tutorial’s examples section.
The main deficiency in these examples is; po.xml and po.xsd is provided instead of the primer.po package. You have to create this package with xjc from po.xml and po.xsd.
C:\Users\ars\Desktop\nbJAXB\modify-marshalARS>xjc po.xsd
parsing a schema...
compiling a schema...
generated\Items.java
generated\ObjectFactory.java
generated\PurchaseOrderType.java
generated\USAddress.java
C:\Users\ars\Desktop\nbJAXB\modify-marshalARS>This command creates a generated dir in the same location as po.xml. You can copy its content to the primer.po package. You may also use the parameters of the xjc command to do this directly.
The directory structure looks like this:

The NetBeans project directory looks like this:
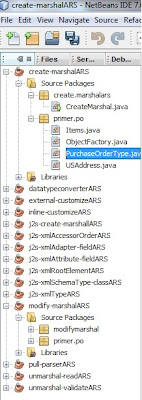
createMarshall.java
package create.marshalars;
//1.The <JWSDP_HOME>/jaxb/samples/create-marshal/
//Main.java class declares imports for four standard Java classes plus three
//JAXB binding framework classes and the primer.po package:
import java.io.IOException;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.Calendar;
import java.util.List;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
import javax.xml.datatype.XMLGregorianCalendar;
import primer.po.*;
public class CreateMarshal {
public static void main(String[] args) {
try {
// 1.A JAXBContext instance is created for handling classes generated in primer.po.
JAXBContext jc = JAXBContext.newInstance("primer.po");
//1.The ObjectFactory class is used to instantiate a new empty PurchaseOrder object.
// creating the ObjectFactory
ObjectFactory objFactory = new ObjectFactory();
// create an empty PurchaseOrder
PurchaseOrderType po = objFactory.createPurchaseOrderType();
//1.Per the constraints in the po.xsd schema, the PurchaseOrder object requires a value for the orderDate attribute. To satisfy this constraint, the orderDate is set using the standard Calendar.getInstance() method from java.util.Calendar.
po.setOrderDate(Calendar.getInstance());
//1.The ObjectFactory is used to instantiate new empty USAddress objects, and the required attributes are set.
USAddress shipTo = createUSAddress(objFactory, "Alice Smith",
"123 Maple Street",
"Cambridge",
"MA",
"12345");
po.setShipTo(shipTo);
USAddress billTo = createUSAddress(objFactory, "Robert Smith",
"8 Oak Avenue",
"Cambridge",
"MA",
"12345");
po.setBillTo(billTo);
//1.The ObjectFactory class is used to instantiate a new empty Items object.
Items items = objFactory.createItems();
//1.A get method is used to get a reference to the ItemType list.
List itemList = items.getItem();
//1.ItemType objects are created and added to the Items list.
itemList.add(createItemType(
objFactory,
"Nosferatu - Special Edition (1929)",
new BigInteger("5"),
new BigDecimal("19.99"),
null,
null,
"242-NO"));
itemList.add(createItemType(objFactory, "The Mummy (1959)",
new BigInteger("3"),
new BigDecimal("19.98"),
null,
null,
"242-MU"));
itemList.add(createItemType(objFactory,
"Godzilla and Mothra: Battle for Earth/Godzilla vs. King Ghidora",
new BigInteger("3"),
new BigDecimal("27.95"),
null,
null,
"242-GZ"));
//1.The items object now contains a list of ItemType objects and can be added to the po object.
po.setItems(items);
//1.A Marshaller instance is created, and the updated XML content is marshalled to system.out. The setProperty API is used to specify output encoding; in this case formatted (human readable) XML format.
Marshaller m = jc.createMarshaller();
m.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, Boolean.TRUE);
m.marshal(po, System.out);
//1.Basic error handling is implemented.
} catch (JAXBException je) {
je.printStackTrace();
}
}
//1.An empty USAddress object is created and its properties set to comply with the schema constraints.
public static USAddress createUSAddress(
ObjectFactory objFactory,
String name,
String street,
String city,
String state,
String zip)
throws JAXBException {
// create an empty USAddress objects
USAddress address = objFactory.createUSAddress();
// set properties on it
address.setName(name);
address.setStreet(street);
address.setCity(city);
address.setState(state);
address.setZip(new BigDecimal(zip));
// return it
return address;
}
//1.Similar to the previous step, an empty ItemType object is created and its properties set to comply with the schema constraints.
public static Items.Item createItemType(ObjectFactory objFactory,
String productName,
BigInteger quantity,
BigDecimal price,
String comment,
Calendar shipDate,
String partNum)
throws JAXBException {
// create an empty ItemType object
Items.Item itemType =
objFactory.createItemsItem();
// set properties on it
itemType.setProductName(productName);
itemType.setQuantity(quantity);
itemType.setUSPrice(price);
itemType.setComment(comment);
itemType.setShipDate(shipDate);
itemType.setPartNum(partNum);
// return it
return itemType;
}
}
Primer.po/Items.java
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, vJAXB 2.1.10 in JDK 6
// See <a href="http://java.sun.com/xml/jaxb">http://java.sun.com/xml/jaxb</a>
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2012.03.06 at 05:03:34 PM EET
//
package primer.po;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
/**
* <p>Java class for Items complex type.
*
* <p>The following schema fragment specifies the expected content contained within this class.
*
* <pre>
* <complexType name="Items">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="item" maxOccurs="unbounded">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="productName" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="quantity">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}positiveInteger">
* <maxExclusive value="100"/>
* </restriction>
* </simpleType>
* </element>
* <element name="USPrice" type="{http://www.w3.org/2001/XMLSchema}decimal"/>
* <element ref="{}comment" minOccurs="0"/>
* <element name="shipDate" type="{http://www.w3.org/2001/XMLSchema}date" minOccurs="0"/>
* </sequence>
* <attribute name="partNum" use="required" type="{}SKU" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </pre>
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Items", propOrder = {
"item"
})
public class Items {
@XmlElement(required = true)
protected List<Items.Item> item;
/**
* Gets the value of the item property.
*
* <p>
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a <CODE>set</CODE> method for the item property.
*
* <p>
* For example, to add a new item, do as follows:
* <pre>
* getItem().add(newItem);
* </pre>
*
*
* <p>
* Objects of the following type(s) are allowed in the list
* {@link Items.Item }
*
*
*/
public List<Items.Item> getItem() {
if (item == null) {
item = new ArrayList<Items.Item>();
}
return this.item;
}
/**
* <p>Java class for anonymous complex type.
*
* <p>The following schema fragment specifies the expected content contained within this class.
*
* <pre>
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="productName" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="quantity">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}positiveInteger">
* <maxExclusive value="100"/>
* </restriction>
* </simpleType>
* </element>
* <element name="USPrice" type="{http://www.w3.org/2001/XMLSchema}decimal"/>
* <element ref="{}comment" minOccurs="0"/>
* <element name="shipDate" type="{http://www.w3.org/2001/XMLSchema}date" minOccurs="0"/>
* </sequence>
* <attribute name="partNum" use="required" type="{}SKU" />
* </restriction>
* </complexContent>
* </complexType>
* </pre>
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"productName",
"quantity",
"usPrice",
"comment",
"shipDate"
})
public static class Item {
@XmlElement(required = true)
protected String productName;
protected BigInteger quantity;
@XmlElement(name = "USPrice", required = true)
protected BigDecimal usPrice;
protected String comment;
@XmlSchemaType(name = "date")
protected Calendar shipDate;
@XmlAttribute(required = true)
protected String partNum;
/**
* Gets the value of the productName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getProductName() {
return productName;
}
/**
* Sets the value of the productName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setProductName(String value) {
this.productName = value;
}
/**
* Gets the value of the quantity property.
*
*/
public BigInteger getQuantity() {
return quantity;
}
/**
* Sets the value of the quantity property.
*
*/
public void setQuantity(BigInteger value) {
this.quantity = value;
}
/**
* Gets the value of the usPrice property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getUSPrice() {
return usPrice;
}
/**
* Sets the value of the usPrice property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setUSPrice(BigDecimal value) {
this.usPrice = value;
}
/**
* Gets the value of the comment property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getComment() {
return comment;
}
/**
* Sets the value of the comment property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setComment(String value) {
this.comment = value;
}
/**
* Gets the value of the shipDate property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public Calendar getShipDate() {
return shipDate;
}
/**
* Sets the value of the shipDate property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setShipDate(Calendar value) {
this.shipDate = value;
}
/**
* Gets the value of the partNum property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPartNum() {
return partNum;
}
/**
* Sets the value of the partNum property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPartNum(String value) {
this.partNum = value;
}
}
}
Primer.po/ObjectFactory.java
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, vJAXB 2.1.10 in JDK 6
// See <a href="http://java.sun.com/xml/jaxb">http://java.sun.com/xml/jaxb</a>
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2012.03.06 at 05:03:34 PM EET
//
package primer.po;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the generated package.
* <p>An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _PurchaseOrder_QNAME = new QName("", "purchaseOrder");
private final static QName _Comment_QNAME = new QName("", "comment");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: generated
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link Items.Item }
*
*/
public Items.Item createItemsItem() {
return new Items.Item();
}
/**
* Create an instance of {@link PurchaseOrderType }
*
*/
public PurchaseOrderType createPurchaseOrderType() {
return new PurchaseOrderType();
}
/**
* Create an instance of {@link Items }
*
*/
public Items createItems() {
return new Items();
}
/**
* Create an instance of {@link USAddress }
*
*/
public USAddress createUSAddress() {
return new USAddress();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link PurchaseOrderType }{@code >}}
*
*/
@XmlElementDecl(namespace = "", name = "purchaseOrder")
public JAXBElement<PurchaseOrderType> createPurchaseOrder(PurchaseOrderType value) {
return new JAXBElement<PurchaseOrderType>(_PurchaseOrder_QNAME, PurchaseOrderType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}}
*
*/
@XmlElementDecl(namespace = "", name = "comment")
public JAXBElement<String> createComment(String value) {
return new JAXBElement<String>(_Comment_QNAME, String.class, null, value);
}
}
Primer.po/PurchaseOrderType.java
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, vJAXB 2.1.10 in JDK 6
// See <a href="http://java.sun.com/xml/jaxb">http://java.sun.com/xml/jaxb</a>
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2012.03.06 at 05:03:34 PM EET
//
package primer.po;
import java.util.Calendar;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
/**
* <p>Java class for PurchaseOrderType complex type.
*
* <p>The following schema fragment specifies the expected content contained within this class.
*
* <pre>
* <complexType name="PurchaseOrderType">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="shipTo" type="{}USAddress"/>
* <element name="billTo" type="{}USAddress"/>
* <element ref="{}comment" minOccurs="0"/>
* <element name="items" type="{}Items"/>
* </sequence>
* <attribute name="orderDate" type="{http://www.w3.org/2001/XMLSchema}date" />
* </restriction>
* </complexContent>
* </complexType>
* </pre>
*
*
*/
@XmlRootElement
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PurchaseOrderType", propOrder = {
"shipTo",
"billTo",
"comment",
"items"
})
public class PurchaseOrderType {
@XmlElement(required = true)
protected USAddress shipTo;
@XmlElement(required = true)
protected USAddress billTo;
protected String comment;
@XmlElement(required = true)
protected Items items;
@XmlAttribute
@XmlSchemaType(name = "date")
protected Calendar orderDate;
/**
* Gets the value of the shipTo property.
*
* @return
* possible object is
* {@link USAddress }
*
*/
public USAddress getShipTo() {
return shipTo;
}
/**
* Sets the value of the shipTo property.
*
* @param value
* allowed object is
* {@link USAddress }
*
*/
public void setShipTo(USAddress value) {
this.shipTo = value;
}
/**
* Gets the value of the billTo property.
*
* @return
* possible object is
* {@link USAddress }
*
*/
public USAddress getBillTo() {
return billTo;
}
/**
* Sets the value of the billTo property.
*
* @param value
* allowed object is
* {@link USAddress }
*
*/
public void setBillTo(USAddress value) {
this.billTo = value;
}
/**
* Gets the value of the comment property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getComment() {
return comment;
}
/**
* Sets the value of the comment property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setComment(String value) {
this.comment = value;
}
/**
* Gets the value of the items property.
*
* @return
* possible object is
* {@link Items }
*
*/
public Items getItems() {
return items;
}
/**
* Sets the value of the items property.
*
* @param value
* allowed object is
* {@link Items }
*
*/
public void setItems(Items value) {
this.items = value;
}
/**
* Gets the value of the orderDate property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public Calendar getOrderDate() {
return orderDate;
}
/**
* Sets the value of the orderDate property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setOrderDate(Calendar value) {
this.orderDate = value;
}
}
Primer.po/USAddress.java
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, vJAXB 2.1.10 in JDK 6
// See <a href="http://java.sun.com/xml/jaxb">http://java.sun.com/xml/jaxb</a>
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2012.03.06 at 05:03:34 PM EET
//
package primer.po;
import java.math.BigDecimal;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* <p>Java class for USAddress complex type.
*
* <p>The following schema fragment specifies the expected content contained within this class.
*
* <pre>
* <complexType name="USAddress">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="name" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="street" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="city" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="state" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="zip" type="{http://www.w3.org/2001/XMLSchema}decimal"/>
* </sequence>
* <attribute name="country" type="{http://www.w3.org/2001/XMLSchema}NMTOKEN" fixed="US" />
* </restriction>
* </complexContent>
* </complexType>
* </pre>
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "USAddress", propOrder = {
"name",
"street",
"city",
"state",
"zip"
})
public class USAddress {
@XmlElement(required = true)
protected String name;
@XmlElement(required = true)
protected String street;
@XmlElement(required = true)
protected String city;
@XmlElement(required = true)
protected String state;
@XmlElement(required = true)
protected BigDecimal zip;
@XmlAttribute
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NMTOKEN")
protected String country;
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the street property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStreet() {
return street;
}
/**
* Sets the value of the street property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStreet(String value) {
this.street = value;
}
/**
* Gets the value of the city property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCity() {
return city;
}
/**
* Sets the value of the city property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCity(String value) {
this.city = value;
}
/**
* Gets the value of the state property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getState() {
return state;
}
/**
* Sets the value of the state property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setState(String value) {
this.state = value;
}
/**
* Gets the value of the zip property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getZip() {
return zip;
}
/**
* Sets the value of the zip property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setZip(BigDecimal value) {
this.zip = value;
}
/**
* Gets the value of the country property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCountry() {
if (country == null) {
return "US";
} else {
return country;
}
}
/**
* Sets the value of the country property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCountry(String value) {
this.country = value;
}
}
Once you create the primer.po package using the xjc command you can use the primer.po in almost all the JAXB examples of JAVAEE5. One problem you may encounter though it may require a root element annotation.
@XmlRootElement
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PurchaseOrderType", propOrder = {
"shipTo",
"billTo",
"comment",
"items"
})
public class PurchaseOrderType {
A better example is given by JAVAEE5 in the j2s-xml-rootElement example:
@XmlRootElement(name = "purchaseOrder")
@XmlType(name = "PurchaseOrderType")
public class PurchaseOrderType {
public CreditCardVendor creditCardVendor;
public USAddress billTo;
public USAddress shipTo;
Do not forget to add:
import javax.xml.bind.annotation.XmlRootElement;
Please feel free to contact me for the working copies of the other examples by e-mail at arsaral(at) yahoo.com
Kind regards.
Ali R+ SARAL
Note. My response will be free of charge and within the same day.
The main deficiency in these examples is; po.xml and po.xsd is provided instead of the primer.po package. You have to create this package with xjc from po.xml and po.xsd.
C:\Users\ars\Desktop\nbJAXB\modify-marshalARS>xjc po.xsd
parsing a schema...
compiling a schema...
generated\Items.java
generated\ObjectFactory.java
generated\PurchaseOrderType.java
generated\USAddress.java
C:\Users\ars\Desktop\nbJAXB\modify-marshalARS>This command creates a generated dir in the same location as po.xml. You can copy its content to the primer.po package. You may also use the parameters of the xjc command to do this directly.
The directory structure looks like this:

The NetBeans project directory looks like this:
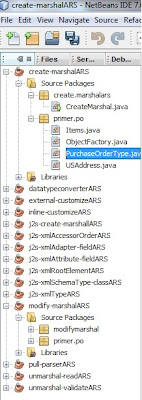
createMarshall.java
package create.marshalars;
//1.The <JWSDP_HOME>/jaxb/samples/create-marshal/
//Main.java class declares imports for four standard Java classes plus three
//JAXB binding framework classes and the primer.po package:
import java.io.IOException;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.Calendar;
import java.util.List;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
import javax.xml.datatype.XMLGregorianCalendar;
import primer.po.*;
public class CreateMarshal {
public static void main(String[] args) {
try {
// 1.A JAXBContext instance is created for handling classes generated in primer.po.
JAXBContext jc = JAXBContext.newInstance("primer.po");
//1.The ObjectFactory class is used to instantiate a new empty PurchaseOrder object.
// creating the ObjectFactory
ObjectFactory objFactory = new ObjectFactory();
// create an empty PurchaseOrder
PurchaseOrderType po = objFactory.createPurchaseOrderType();
//1.Per the constraints in the po.xsd schema, the PurchaseOrder object requires a value for the orderDate attribute. To satisfy this constraint, the orderDate is set using the standard Calendar.getInstance() method from java.util.Calendar.
po.setOrderDate(Calendar.getInstance());
//1.The ObjectFactory is used to instantiate new empty USAddress objects, and the required attributes are set.
USAddress shipTo = createUSAddress(objFactory, "Alice Smith",
"123 Maple Street",
"Cambridge",
"MA",
"12345");
po.setShipTo(shipTo);
USAddress billTo = createUSAddress(objFactory, "Robert Smith",
"8 Oak Avenue",
"Cambridge",
"MA",
"12345");
po.setBillTo(billTo);
//1.The ObjectFactory class is used to instantiate a new empty Items object.
Items items = objFactory.createItems();
//1.A get method is used to get a reference to the ItemType list.
List itemList = items.getItem();
//1.ItemType objects are created and added to the Items list.
itemList.add(createItemType(
objFactory,
"Nosferatu - Special Edition (1929)",
new BigInteger("5"),
new BigDecimal("19.99"),
null,
null,
"242-NO"));
itemList.add(createItemType(objFactory, "The Mummy (1959)",
new BigInteger("3"),
new BigDecimal("19.98"),
null,
null,
"242-MU"));
itemList.add(createItemType(objFactory,
"Godzilla and Mothra: Battle for Earth/Godzilla vs. King Ghidora",
new BigInteger("3"),
new BigDecimal("27.95"),
null,
null,
"242-GZ"));
//1.The items object now contains a list of ItemType objects and can be added to the po object.
po.setItems(items);
//1.A Marshaller instance is created, and the updated XML content is marshalled to system.out. The setProperty API is used to specify output encoding; in this case formatted (human readable) XML format.
Marshaller m = jc.createMarshaller();
m.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, Boolean.TRUE);
m.marshal(po, System.out);
//1.Basic error handling is implemented.
} catch (JAXBException je) {
je.printStackTrace();
}
}
//1.An empty USAddress object is created and its properties set to comply with the schema constraints.
public static USAddress createUSAddress(
ObjectFactory objFactory,
String name,
String street,
String city,
String state,
String zip)
throws JAXBException {
// create an empty USAddress objects
USAddress address = objFactory.createUSAddress();
// set properties on it
address.setName(name);
address.setStreet(street);
address.setCity(city);
address.setState(state);
address.setZip(new BigDecimal(zip));
// return it
return address;
}
//1.Similar to the previous step, an empty ItemType object is created and its properties set to comply with the schema constraints.
public static Items.Item createItemType(ObjectFactory objFactory,
String productName,
BigInteger quantity,
BigDecimal price,
String comment,
Calendar shipDate,
String partNum)
throws JAXBException {
// create an empty ItemType object
Items.Item itemType =
objFactory.createItemsItem();
// set properties on it
itemType.setProductName(productName);
itemType.setQuantity(quantity);
itemType.setUSPrice(price);
itemType.setComment(comment);
itemType.setShipDate(shipDate);
itemType.setPartNum(partNum);
// return it
return itemType;
}
}
Primer.po/Items.java
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, vJAXB 2.1.10 in JDK 6
// See <a href="http://java.sun.com/xml/jaxb">http://java.sun.com/xml/jaxb</a>
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2012.03.06 at 05:03:34 PM EET
//
package primer.po;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
/**
* <p>Java class for Items complex type.
*
* <p>The following schema fragment specifies the expected content contained within this class.
*
* <pre>
* <complexType name="Items">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="item" maxOccurs="unbounded">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="productName" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="quantity">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}positiveInteger">
* <maxExclusive value="100"/>
* </restriction>
* </simpleType>
* </element>
* <element name="USPrice" type="{http://www.w3.org/2001/XMLSchema}decimal"/>
* <element ref="{}comment" minOccurs="0"/>
* <element name="shipDate" type="{http://www.w3.org/2001/XMLSchema}date" minOccurs="0"/>
* </sequence>
* <attribute name="partNum" use="required" type="{}SKU" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </pre>
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Items", propOrder = {
"item"
})
public class Items {
@XmlElement(required = true)
protected List<Items.Item> item;
/**
* Gets the value of the item property.
*
* <p>
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a <CODE>set</CODE> method for the item property.
*
* <p>
* For example, to add a new item, do as follows:
* <pre>
* getItem().add(newItem);
* </pre>
*
*
* <p>
* Objects of the following type(s) are allowed in the list
* {@link Items.Item }
*
*
*/
public List<Items.Item> getItem() {
if (item == null) {
item = new ArrayList<Items.Item>();
}
return this.item;
}
/**
* <p>Java class for anonymous complex type.
*
* <p>The following schema fragment specifies the expected content contained within this class.
*
* <pre>
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="productName" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="quantity">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}positiveInteger">
* <maxExclusive value="100"/>
* </restriction>
* </simpleType>
* </element>
* <element name="USPrice" type="{http://www.w3.org/2001/XMLSchema}decimal"/>
* <element ref="{}comment" minOccurs="0"/>
* <element name="shipDate" type="{http://www.w3.org/2001/XMLSchema}date" minOccurs="0"/>
* </sequence>
* <attribute name="partNum" use="required" type="{}SKU" />
* </restriction>
* </complexContent>
* </complexType>
* </pre>
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"productName",
"quantity",
"usPrice",
"comment",
"shipDate"
})
public static class Item {
@XmlElement(required = true)
protected String productName;
protected BigInteger quantity;
@XmlElement(name = "USPrice", required = true)
protected BigDecimal usPrice;
protected String comment;
@XmlSchemaType(name = "date")
protected Calendar shipDate;
@XmlAttribute(required = true)
protected String partNum;
/**
* Gets the value of the productName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getProductName() {
return productName;
}
/**
* Sets the value of the productName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setProductName(String value) {
this.productName = value;
}
/**
* Gets the value of the quantity property.
*
*/
public BigInteger getQuantity() {
return quantity;
}
/**
* Sets the value of the quantity property.
*
*/
public void setQuantity(BigInteger value) {
this.quantity = value;
}
/**
* Gets the value of the usPrice property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getUSPrice() {
return usPrice;
}
/**
* Sets the value of the usPrice property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setUSPrice(BigDecimal value) {
this.usPrice = value;
}
/**
* Gets the value of the comment property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getComment() {
return comment;
}
/**
* Sets the value of the comment property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setComment(String value) {
this.comment = value;
}
/**
* Gets the value of the shipDate property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public Calendar getShipDate() {
return shipDate;
}
/**
* Sets the value of the shipDate property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setShipDate(Calendar value) {
this.shipDate = value;
}
/**
* Gets the value of the partNum property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPartNum() {
return partNum;
}
/**
* Sets the value of the partNum property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPartNum(String value) {
this.partNum = value;
}
}
}
Primer.po/ObjectFactory.java
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, vJAXB 2.1.10 in JDK 6
// See <a href="http://java.sun.com/xml/jaxb">http://java.sun.com/xml/jaxb</a>
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2012.03.06 at 05:03:34 PM EET
//
package primer.po;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlElementDecl;
import javax.xml.bind.annotation.XmlRegistry;
import javax.xml.namespace.QName;
/**
* This object contains factory methods for each
* Java content interface and Java element interface
* generated in the generated package.
* <p>An ObjectFactory allows you to programatically
* construct new instances of the Java representation
* for XML content. The Java representation of XML
* content can consist of schema derived interfaces
* and classes representing the binding of schema
* type definitions, element declarations and model
* groups. Factory methods for each of these are
* provided in this class.
*
*/
@XmlRegistry
public class ObjectFactory {
private final static QName _PurchaseOrder_QNAME = new QName("", "purchaseOrder");
private final static QName _Comment_QNAME = new QName("", "comment");
/**
* Create a new ObjectFactory that can be used to create new instances of schema derived classes for package: generated
*
*/
public ObjectFactory() {
}
/**
* Create an instance of {@link Items.Item }
*
*/
public Items.Item createItemsItem() {
return new Items.Item();
}
/**
* Create an instance of {@link PurchaseOrderType }
*
*/
public PurchaseOrderType createPurchaseOrderType() {
return new PurchaseOrderType();
}
/**
* Create an instance of {@link Items }
*
*/
public Items createItems() {
return new Items();
}
/**
* Create an instance of {@link USAddress }
*
*/
public USAddress createUSAddress() {
return new USAddress();
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link PurchaseOrderType }{@code >}}
*
*/
@XmlElementDecl(namespace = "", name = "purchaseOrder")
public JAXBElement<PurchaseOrderType> createPurchaseOrder(PurchaseOrderType value) {
return new JAXBElement<PurchaseOrderType>(_PurchaseOrder_QNAME, PurchaseOrderType.class, null, value);
}
/**
* Create an instance of {@link JAXBElement }{@code <}{@link String }{@code >}}
*
*/
@XmlElementDecl(namespace = "", name = "comment")
public JAXBElement<String> createComment(String value) {
return new JAXBElement<String>(_Comment_QNAME, String.class, null, value);
}
}
Primer.po/PurchaseOrderType.java
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, vJAXB 2.1.10 in JDK 6
// See <a href="http://java.sun.com/xml/jaxb">http://java.sun.com/xml/jaxb</a>
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2012.03.06 at 05:03:34 PM EET
//
package primer.po;
import java.util.Calendar;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
/**
* <p>Java class for PurchaseOrderType complex type.
*
* <p>The following schema fragment specifies the expected content contained within this class.
*
* <pre>
* <complexType name="PurchaseOrderType">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="shipTo" type="{}USAddress"/>
* <element name="billTo" type="{}USAddress"/>
* <element ref="{}comment" minOccurs="0"/>
* <element name="items" type="{}Items"/>
* </sequence>
* <attribute name="orderDate" type="{http://www.w3.org/2001/XMLSchema}date" />
* </restriction>
* </complexContent>
* </complexType>
* </pre>
*
*
*/
@XmlRootElement
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PurchaseOrderType", propOrder = {
"shipTo",
"billTo",
"comment",
"items"
})
public class PurchaseOrderType {
@XmlElement(required = true)
protected USAddress shipTo;
@XmlElement(required = true)
protected USAddress billTo;
protected String comment;
@XmlElement(required = true)
protected Items items;
@XmlAttribute
@XmlSchemaType(name = "date")
protected Calendar orderDate;
/**
* Gets the value of the shipTo property.
*
* @return
* possible object is
* {@link USAddress }
*
*/
public USAddress getShipTo() {
return shipTo;
}
/**
* Sets the value of the shipTo property.
*
* @param value
* allowed object is
* {@link USAddress }
*
*/
public void setShipTo(USAddress value) {
this.shipTo = value;
}
/**
* Gets the value of the billTo property.
*
* @return
* possible object is
* {@link USAddress }
*
*/
public USAddress getBillTo() {
return billTo;
}
/**
* Sets the value of the billTo property.
*
* @param value
* allowed object is
* {@link USAddress }
*
*/
public void setBillTo(USAddress value) {
this.billTo = value;
}
/**
* Gets the value of the comment property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getComment() {
return comment;
}
/**
* Sets the value of the comment property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setComment(String value) {
this.comment = value;
}
/**
* Gets the value of the items property.
*
* @return
* possible object is
* {@link Items }
*
*/
public Items getItems() {
return items;
}
/**
* Sets the value of the items property.
*
* @param value
* allowed object is
* {@link Items }
*
*/
public void setItems(Items value) {
this.items = value;
}
/**
* Gets the value of the orderDate property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public Calendar getOrderDate() {
return orderDate;
}
/**
* Sets the value of the orderDate property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setOrderDate(Calendar value) {
this.orderDate = value;
}
}
Primer.po/USAddress.java
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, vJAXB 2.1.10 in JDK 6
// See <a href="http://java.sun.com/xml/jaxb">http://java.sun.com/xml/jaxb</a>
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2012.03.06 at 05:03:34 PM EET
//
package primer.po;
import java.math.BigDecimal;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* <p>Java class for USAddress complex type.
*
* <p>The following schema fragment specifies the expected content contained within this class.
*
* <pre>
* <complexType name="USAddress">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="name" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="street" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="city" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="state" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="zip" type="{http://www.w3.org/2001/XMLSchema}decimal"/>
* </sequence>
* <attribute name="country" type="{http://www.w3.org/2001/XMLSchema}NMTOKEN" fixed="US" />
* </restriction>
* </complexContent>
* </complexType>
* </pre>
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "USAddress", propOrder = {
"name",
"street",
"city",
"state",
"zip"
})
public class USAddress {
@XmlElement(required = true)
protected String name;
@XmlElement(required = true)
protected String street;
@XmlElement(required = true)
protected String city;
@XmlElement(required = true)
protected String state;
@XmlElement(required = true)
protected BigDecimal zip;
@XmlAttribute
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlSchemaType(name = "NMTOKEN")
protected String country;
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the street property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStreet() {
return street;
}
/**
* Sets the value of the street property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStreet(String value) {
this.street = value;
}
/**
* Gets the value of the city property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCity() {
return city;
}
/**
* Sets the value of the city property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCity(String value) {
this.city = value;
}
/**
* Gets the value of the state property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getState() {
return state;
}
/**
* Sets the value of the state property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setState(String value) {
this.state = value;
}
/**
* Gets the value of the zip property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getZip() {
return zip;
}
/**
* Sets the value of the zip property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setZip(BigDecimal value) {
this.zip = value;
}
/**
* Gets the value of the country property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCountry() {
if (country == null) {
return "US";
} else {
return country;
}
}
/**
* Sets the value of the country property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCountry(String value) {
this.country = value;
}
}
Once you create the primer.po package using the xjc command you can use the primer.po in almost all the JAXB examples of JAVAEE5. One problem you may encounter though it may require a root element annotation.
@XmlRootElement
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "PurchaseOrderType", propOrder = {
"shipTo",
"billTo",
"comment",
"items"
})
public class PurchaseOrderType {
A better example is given by JAVAEE5 in the j2s-xml-rootElement example:
@XmlRootElement(name = "purchaseOrder")
@XmlType(name = "PurchaseOrderType")
public class PurchaseOrderType {
public CreditCardVendor creditCardVendor;
public USAddress billTo;
public USAddress shipTo;
Do not forget to add:
import javax.xml.bind.annotation.XmlRootElement;
Please feel free to contact me for the working copies of the other examples by e-mail at arsaral(at) yahoo.com
Kind regards.
Ali R+ SARAL
Note. My response will be free of charge and within the same day.
Subscribe to:
Posts (Atom)