1.1 Create an Enterprise Application (name=ServletStatelessARSEAR)
1.2 Say OK to create an ejbClient (name = ServletStatelessARS-ejbClient)
1.3 Leave the rest as it is, you will add the EJB and war projects later on.
As seen in the picture.
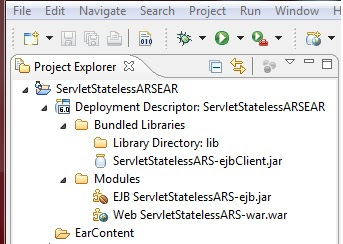
2.1 Create under ejbModule an interface file at the ServletStatelessARS-ejbClient client application (loc/name=enterpriseARS.servlet_stateless_ejbClient/StatelessSessionARS.java)
package enterpriseARS.servlet_stateless_ejbClient;
public interface StatelessSessionARS {
public String sayHelloARS(String name);
public int add2Parms(int parm1, int parm2);
}
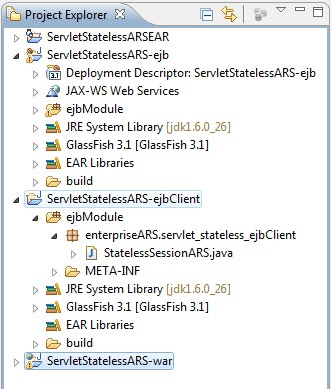
2.2 Create an EJB application (name=ServletStatelessARS-ejb) and attach it to the Enterprise application using the related creation option.
2.3 Create under ejbModule (loc/name=enterpriseARS.servlet_stateless_ejb/StatelessSessionARSBean.java)
package enterpriseARS.servlet_stateless_ejb;
import javax.ejb.LocalBean;
import javax.ejb.Stateless;
import javax.jws.WebService;
import enterpriseARS.servlet_stateless_ejbClient.StatelessSessionARS;
@WebService (only for diagnostic purposes, to be used for Glassfish endpoint testing)
@Stateless
public class StatelessSessionARSBean
implements StatelessSessionARS {
public String sayHelloARS(String name) {
return "HelloARS, " + name + "!\n";
}
public int add2Parms(int parm1, int parm2){
return(parm1+parm2);
}
}
3.1 Create a dynamic web application (name=ServletStatelessARS-war) and attach it to the Enterprise application using the related creation option.
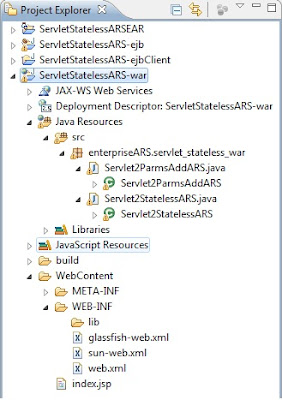
3.2 Web/index.jsp should be:
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"
"http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>GlassFish JSP Page</title>
</head>
<body>
<h1>Calculator Service</h1>
<form name="Submit" action="Servlet2ParmsAddARS">
<input type="text" name="value1" value="2" size="3"/>+
<input type="text" name="value2" value="2" size="3"/>=
<input type="submit" value="Get Result" name="getResult" />
</form>
</body>
</html>
3.3 Web.xml should be:
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.5" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd">
<display-name>ServletStatelessARS</display-name>
<distributable/>
<servlet>
<servlet-name>Servlet2StatelessARS</servlet-name>
<servlet-class>enterpriseARS.servlet_stateless_war.Servlet2StatelessARS</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>Servlet2StatelessARS</servlet-name>
<url-pattern>/servlet</url-pattern>
</servlet-mapping>
<servlet>
<servlet-name>Servlet2ParmsAddARS</servlet-name>
<servlet-class>enterpriseARS.servlet_stateless_war.Servlet2ParmsAddARS</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>Servlet2ParmsAddARS</servlet-name>
<url-pattern>/Servlet2ParmsAddARS</url-pattern>
</servlet-mapping>
<welcome-file-list>
<welcome-file>
index.jsp
servlet
</welcome-file>
</welcome-file-list>
</web-app>
3.4 In the Java Resources/src/enterpriseARS.servlet_stateless_war package,
Create Servlet2ParmsAddARS.java
package enterpriseARS.servlet_stateless_war;
import java.io.*;
import javax.ejb.EJB;
import javax.servlet.*;
import javax.servlet.http.*;
import javax.naming.*;
import enterpriseARS.servlet_stateless_ejbClient.*;
// Though it is perfectly fine to declare the dependency on the bean
// at the type level, it is not required for stateless session bean
// Hence the next two lines are commented and we rely on the
// container to inject the bean.
// @EJB(name="StatelessSession", beanInterface=StatelessSession.class)
public class Servlet2ParmsAddARS
extends HttpServlet {
// Using injection for Stateless session bean is still thread-safe since
// the ejb container will route every request to different
// bean instances. However, for Stateful session beans the
// dependency on the bean must be declared at the type level
@EJB
private StatelessSessionARS sless;
public void service(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
resp.setContentType("text/html");
PrintWriter out = resp.getWriter();
try {
out.println("<h2>Servlet ClientServlet at " + req.getContextPath () + "</h2>");
int i = Integer.parseInt(req.getParameter("value1"));
int j = Integer.parseInt(req.getParameter("value2"));
int result = sless.add2Parms(i,j);
out.println("<br/>");
out.println("Result:");
out.println("" + i + " + " + j + " = " + result);
} catch (Exception ex) {
ex.printStackTrace();
System.out.println("webclient servlet test failed");
throw new ServletException(ex);
}
}
}
3.5 create Servlet2StatelessARS.java at the same package location.
package enterpriseARS.servlet_stateless_war;
import java.io.*;
import javax.ejb.EJB;
import javax.servlet.*;
import javax.servlet.http.*;
import javax.naming.*;
import enterpriseARS.servlet_stateless_ejbClient.*;
public class Servlet2StatelessARS
extends HttpServlet {
@EJB
private StatelessSessionARS sless;
public void service(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
resp.setContentType("text/html");
PrintWriter out = resp.getWriter();
try {
out.println("<HTML> <HEAD> <TITLE> Servlet Output </TITLE> </HEAD> <BODY BGCOLOR=white>");
out.println("<CENTER> <FONT size=+1> Servlet2Stateless:: Please enter your name </FONT> </CENTER> <p> ");
out.println("<form method=\"POST\">");
out.println("<TABLE>");
out.println("<tr><td>Name: </td>");
out.println("<td><input type=\"text\" name=\"name\"> </td>");
out.println("</tr><tr><td></td>");
out.println("<td><input type=\"submit\" name=\"sub\"> </td>");
out.println("</tr>");
out.println("</TABLE>");
out.println("</form>");
String val = req.getParameter("name");
if ((val != null) && (val.trim().length() > 0)) {
out
.println("<FONT size=+1 color=red> Greeting from StatelessSessionBean: </FONT> "
+ sless.sayHelloARS(val) + "<br>");
}
out.println("</BODY> </HTML> ");
} catch (Exception ex) {
ex.printStackTrace();
System.out.println("webclient servlet test failed");
throw new ServletException(ex);
}
}
}
4.1 It will give IDE and compile error for the EJB because currently the dynamic web application does not see the EJB application.
4.2 Right click on the dynamic web application and check that Project references indicates ejb and ejbClient applications as referred.
4.3 Go to the Java Build Path and click on the Projects tab. Add the ejb and ejbClient applications.
4.4 The IDE – compile error for the EJB disappears.
5. You may also check yhe ear application for the same items but they are done automatically for it.
6. Make sure you test the application in an orderly manner. Build the EAR application and also build the others if necessary. When you run the war application the calculator works as default. If you run
http://localhost:8080/ServletStatelessARS-war/servlet then the hello message works.
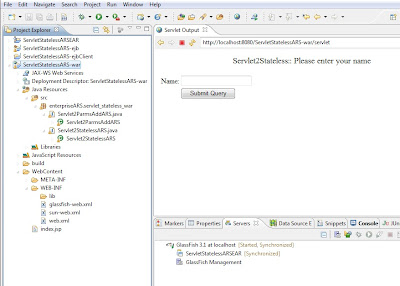
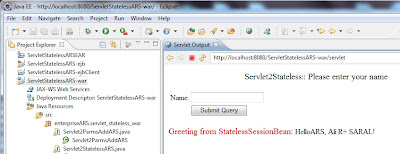
7. If you experience any problems export the ear application to a war file at the
C:\Program Files\glassfish-3.1\glassfish\domains\domain1\autodeploy
Autodeploy directory of Glassfish. Then restart Glassfish, everything will be OK.
It is free of charge to request a copy of the source files. I am sometimes hecticly busy but I promise to respond in a couple of hours.
Cheers.
Ali R+ SARAL